c++使用gdi+将bmp图片发送到打印机打印
时间: 2024-05-03 20:23:17 浏览: 12
要使用GDI将BMP图像发送到打印机打印,您需要执行以下步骤:
1. 打开打印机设备上下文(DC):
```
HDC hdcPrinter = CreateDC("WINSPOOL", printerName, NULL, NULL);
```
其中,`printerName`是您要打印的打印机的名称。
2. 创建一个内存DC,并将BMP图像绘制到该DC上:
```
HDC hdcMem = CreateCompatibleDC(NULL);
HBITMAP hBitmap = (HBITMAP)LoadImage(NULL, bmpFileName, IMAGE_BITMAP, 0, 0, LR_LOADFROMFILE);
HBITMAP hOldBitmap = (HBITMAP)SelectObject(hdcMem, hBitmap);
BITMAP bm;
GetObject(hBitmap, sizeof(BITMAP), &bm);
BitBlt(hdcMem, 0, 0, bm.bmWidth, bm.bmHeight, hdcMem, 0, 0, SRCCOPY);
```
其中,`bmpFileName`是BMP图像文件的名称。
3. 获取打印机DC的打印机信息:
```
PRINTER_INFO_2 printerInfo;
DWORD dwBytesNeeded;
GetPrinter(hdcPrinter, 2, NULL, 0, &dwBytesNeeded);
BYTE* pBuffer = new BYTE[dwBytesNeeded];
GetPrinter(hdcPrinter, 2, pBuffer, dwBytesNeeded, &dwBytesNeeded);
memcpy(&printerInfo, pBuffer, sizeof(PRINTER_INFO_2));
delete[] pBuffer;
```
4. 创建一个打印机DC,并将其与打印机连接:
```
HDC hdcPrint = CreateDC(NULL, printerInfo.pPrinterName, NULL, NULL);
```
5. 设置打印机DC的打印机模式,并将内存DC中的BMP图像打印到打印机DC上:
```
DOCINFO di;
ZeroMemory(&di, sizeof(DOCINFO));
di.cbSize = sizeof(DOCINFO);
StartDoc(hdcPrint, &di);
StartPage(hdcPrint);
SetMapMode(hdcPrint, MM_ANISOTROPIC);
SetViewportExtEx(hdcPrint, bm.bmWidth, bm.bmHeight, NULL);
SetWindowExtEx(hdcPrint, bm.bmWidth, bm.bmHeight, NULL);
BitBlt(hdcPrint, 0, 0, bm.bmWidth, bm.bmHeight, hdcMem, 0, 0, SRCCOPY);
EndPage(hdcPrint);
EndDoc(hdcPrint);
```
6. 释放所有DC和位图:
```
SelectObject(hdcMem, hOldBitmap);
DeleteDC(hdcMem);
DeleteDC(hdcPrint);
DeleteObject(hBitmap);
```
完整的代码示例:
```
void PrintBmpOnPrinter(LPCTSTR printerName, LPCTSTR bmpFileName)
{
HDC hdcPrinter = CreateDC("WINSPOOL", printerName, NULL, NULL);
HDC hdcMem = CreateCompatibleDC(NULL);
HBITMAP hBitmap = (HBITMAP)LoadImage(NULL, bmpFileName, IMAGE_BITMAP, 0, 0, LR_LOADFROMFILE);
HBITMAP hOldBitmap = (HBITMAP)SelectObject(hdcMem, hBitmap);
BITMAP bm;
GetObject(hBitmap, sizeof(BITMAP), &bm);
BitBlt(hdcMem, 0, 0, bm.bmWidth, bm.bmHeight, hdcMem, 0, 0, SRCCOPY);
PRINTER_INFO_2 printerInfo;
DWORD dwBytesNeeded;
GetPrinter(hdcPrinter, 2, NULL, 0, &dwBytesNeeded);
BYTE* pBuffer = new BYTE[dwBytesNeeded];
GetPrinter(hdcPrinter, 2, pBuffer, dwBytesNeeded, &dwBytesNeeded);
memcpy(&printerInfo, pBuffer, sizeof(PRINTER_INFO_2));
delete[] pBuffer;
HDC hdcPrint = CreateDC(NULL, printerInfo.pPrinterName, NULL, NULL);
DOCINFO di;
ZeroMemory(&di, sizeof(DOCINFO));
di.cbSize = sizeof(DOCINFO);
StartDoc(hdcPrint, &di);
StartPage(hdcPrint);
SetMapMode(hdcPrint, MM_ANISOTROPIC);
SetViewportExtEx(hdcPrint, bm.bmWidth, bm.bmHeight, NULL);
SetWindowExtEx(hdcPrint, bm.bmWidth, bm.bmHeight, NULL);
BitBlt(hdcPrint, 0, 0, bm.bmWidth, bm.bmHeight, hdcMem, 0, 0, SRCCOPY);
EndPage(hdcPrint);
EndDoc(hdcPrint);
SelectObject(hdcMem, hOldBitmap);
DeleteDC(hdcMem);
DeleteDC(hdcPrint);
DeleteObject(hBitmap);
}
```
相关推荐
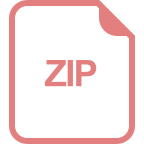














