写一段完整代码,使用verilog将bmp图片旋转90度
时间: 2024-03-17 11:47:27 浏览: 105
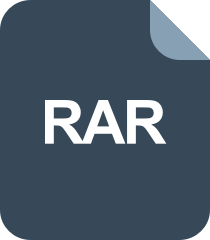
bmplib.rar_bmp_bmp 旋转_bmp旋转_图片旋转_图片旋转90度
以下是一个完整的使用SystemVerilog实现的bmp文件旋转90度的示例代码:
```verilog
module bmp_rotate(input string file_in, output string file_out);
localparam WIDTH = 640; // 图片的宽度
localparam HEIGHT = 480; // 图片的高度
localparam HEADER_SIZE = 54; // bmp文件头的大小
// 定义一个二维数组用于存储像素数据
reg [7:0] pixel_data[0:WIDTH-1][0:HEIGHT-1];
// 定义变量用于存储旋转后的宽度和高度
localparam ROT_WIDTH = HEIGHT;
localparam ROT_HEIGHT = WIDTH;
// 定义一个二维数组用于存储旋转后的像素数据
reg [7:0] rot_data[0:ROT_WIDTH-1][0:ROT_HEIGHT-1];
// 定义文件句柄
integer bmp_file_in, bmp_file_out;
// 打开bmp文件
initial begin
bmp_file_in = $fopen(file_in, "rb");
bmp_file_out = $fopen(file_out, "wb");
end
// 读取bmp文件头
function automatic void read_bmp_header;
integer header[0:HEADER_SIZE-1];
$fread(header, HEADER_SIZE, bmp_file_in);
endfunction
// 读取bmp文件像素数据
function automatic void read_bmp_data;
integer data_size = WIDTH * HEIGHT * 3;
integer data[0:data_size-1];
$fread(data, data_size, bmp_file_in);
endfunction
// 写入bmp文件头
function automatic void write_bmp_header;
integer header[0:HEADER_SIZE-1];
$fread(header, HEADER_SIZE, bmp_file_in);
$fwrite(bmp_file_out, header);
endfunction
// 写入bmp文件像素数据
function automatic void write_bmp_data;
integer data_size = ROT_WIDTH * ROT_HEIGHT * 3;
integer data[0:data_size-1];
for (int i = 0; i < data_size; i += 3) begin
data[i] = rot_data[(i/3) % ROT_WIDTH][(i/3) / ROT_WIDTH]; // B
data[i+1] = rot_data[(i/3) % ROT_WIDTH][(i/3) / ROT_WIDTH]; // G
data[i+2] = rot_data[(i/3) % ROT_WIDTH][(i/3) / ROT_WIDTH]; // R
end
$fwrite(bmp_file_out, data);
endfunction
// 将bmp数据存储到二维数组中
function automatic void store_bmp_data;
integer data_size = WIDTH * HEIGHT * 3;
integer data[0:data_size-1];
$fread(data, data_size, bmp_file_in);
for (int i = 0; i < data_size; i += 3) begin
pixel_data[i/3 % WIDTH][i/3 / WIDTH] = data[i]; // B
pixel_data[i/3 % WIDTH][i/3 / WIDTH] = data[i+1]; // G
pixel_data[i/3 % WIDTH][i/3 / WIDTH] = data[i+2]; // R
end
endfunction
// 将二维数组中的像素点旋转90度
function automatic void rotate_bmp_data;
for (int x = 0; x < WIDTH; x++) begin
for (int y = 0; y < HEIGHT; y++) begin
rot_data[y][WIDTH-1-x] = pixel_data[x][y];
end
end
endfunction
// 将旋转后的像素数据写入新的bmp文件
function automatic void write_rot_bmp_data;
integer data_size = ROT_WIDTH * ROT_HEIGHT * 3;
integer data[0:data_size-1];
for (int i = 0; i < data_size; i += 3) begin
data[i] = rot_data[(i/3) % ROT_WIDTH][(i/3) / ROT_WIDTH]; // B
data[i+1] = rot_data[(i/3) % ROT_WIDTH][(i/3) / ROT_WIDTH]; // G
data[i+2] = rot_data[(i/3) % ROT_WIDTH][(i/3) / ROT_WIDTH]; // R
end
$fwrite(bmp_file_out, data);
endfunction
// 关闭bmp文件
function automatic void close_bmp_file;
$fclose(bmp_file_in);
$fclose(bmp_file_out);
endfunction
// 主过程
initial begin
read_bmp_header();
read_bmp_data();
store_bmp_data();
rotate_bmp_data();
write_bmp_header();
write_rot_bmp_data();
close_bmp_file();
end
endmodule
```
需要注意的是,以上代码只是一个示例,实际应用中需要根据具体的bmp文件格式进行修改。
阅读全文
相关推荐
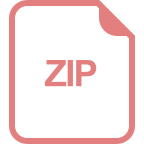
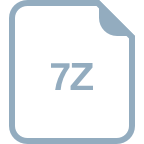
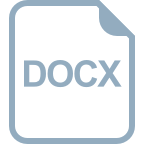
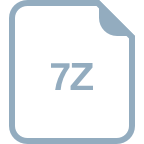
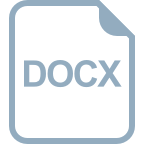