在SV中如何例化二维数组
时间: 2024-01-27 17:16:06 浏览: 142
在SV中,可以使用以下语法来实例化一个二维数组:
```systemverilog
type data_t = int;
data_t my_array[$][10]; // 一个有 $ 行,10 列的二维数组
```
其中,`data_t` 是数组中元素的类型,`$` 表示数组的行数可以根据实际情况动态确定,`10` 表示数组的列数为 10。
你也可以在实例化时初始化数组的值,例如:
```systemverilog
type data_t = int;
data_t my_array[2][3] = '{'{1, 2, 3}, {4, 5, 6}}; // 一个有 2 行 3 列的二维数组,初始值为 {{1, 2, 3}, {4, 5, 6}}
```
这里使用了花括号 `{}` 来表示数组的初始化值,其中每一对花括号表示一行,内部的值表示该行中每个元素的值。
相关问题
在sv中怎么打印二维数组
在SystemVerilog中,可以使用$display或$writef等系统任务来打印二维数组。例如,假设有一个名为matrix的二维整型数组,可以使用以下代码打印数组:
```systemverilog
int matrix[3][3] = '{'{1, 2, 3}, '{4, 5, 6}, '{7, 8, 9}};
for(int i=0; i<3; i++) begin
for(int j=0; j<3; j++) begin
$display("matrix[%0d][%0d] = %0d", i, j, matrix[i][j]);
end
end
```
输出结果为:
```
matrix[0][0] = 1
matrix[0][1] = 2
matrix[0][2] = 3
matrix[1][0] = 4
matrix[1][1] = 5
matrix[1][2] = 6
matrix[2][0] = 7
matrix[2][1] = 8
matrix[2][2] = 9
```
其中,`%0d`是格式说明符,用于表示输出整型数值。使用两重循环来遍历二维数组中的每个元素,`$display`用于打印数组元素的值。
SV用foreach遍历二维数组
在SystemVerilog中,可以使用`foreach`循环来遍历二维数组。以下是一个使用`foreach`循环遍历二维数组的示例代码:
```systemverilog
module test;
reg [7:0] two_dimensional_array [3:0][3:0] = '{ '{8, 7, 6, 5}, '{4, 3, 2, 1}, '{0, 9, 8, 7}, '{6, 5, 4, 3} };
initial begin
foreach (two_dimensional_array[row]) begin
foreach (two_dimensional_array[row][col]) begin
$display("Value at [%0d][%0d]: %0d", row, col, two_dimensional_array[row][col]);
end
end
end
endmodule
```
在上面的代码中,我们定义了一个名为`two_dimensional_array`的二维数组,它是一个4x4的数组。`two_dimensional_array`中包含了一些随机的8位寄存器值。
在`initial`块中,我们使用两个嵌套的`foreach`循环来遍历二维数组。外部的`foreach (two_dimensional_array[row])`循环用于遍历一维数组的每一行。内部的`foreach (two_dimensional_array[row][col])`循环用于遍历一维数组中的每个元素。
在每次循环迭代时,我们使用`$display`系统任务打印出当前元素的行、列和值。
当我们运行这段代码时,它会输出如下内容:
```
Value at [0][0]: 8
Value at [0][1]: 7
Value at [0][2]: 6
Value at [0][3]: 5
Value at [1][0]: 4
Value at [1][1]: 3
Value at [1][2]: 2
Value at [1][3]: 1
Value at [2][0]: 0
Value at [2][1]: 9
Value at [2][2]: 8
Value at [2][3]: 7
Value at [3][0]: 6
Value at [3][1]: 5
Value at [3][2]: 4
Value at [3][3]: 3
```
这个例子演示了如何使用`foreach`循环遍历二维数组,并打印出每个元素的位置和值。希望对您有所帮助!如果您有其他问题,请随时提问。
阅读全文
相关推荐
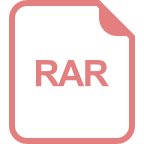










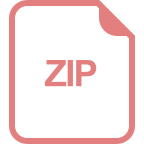


