基于情感词典对excel表格中的在线评论进行情感分析
时间: 2023-08-12 15:03:29 浏览: 220
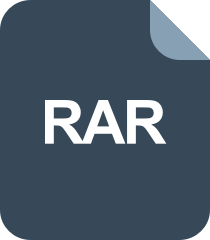
基于情感词典的情感分析
在Python中,可以使用pandas库读取Excel表格数据,使用情感词典对评论进行情感分析。具体步骤如下:
1.安装必要的Python库。可以使用以下命令安装pandas和jieba库:
```
pip install pandas
pip install jieba
```
2.读取Excel表格数据。使用pandas库的read_excel函数读取Excel表格数据:
```python
import pandas as pd
# 读取Excel文件
df = pd.read_excel('comments.xlsx')
```
其中,'comments.xlsx'是Excel文件的文件名。
3.对评论进行分词。使用jieba库对评论进行分词:
```python
import jieba
# 对评论进行分词
df['comment_cut'] = df['comment'].apply(lambda x: ' '.join(jieba.cut(x)))
```
其中,'comment'是Excel表格中评论所在列的列名,'comment_cut'是新建的一列,用于存储分词后的评论。
4.加载情感词典。可以使用Python的字典类型来存储情感词典:
```python
# 加载情感词典
sentiment_dict = {}
with open('sentiment_dict.txt', 'r', encoding='utf-8') as f:
for line in f:
word, score = line.strip().split('\t')
sentiment_dict[word] = float(score)
```
其中,'sentiment_dict.txt'是情感词典文件的文件名,每行格式为“词语\t情感得分”。
5.计算评论的情感得分。可以使用pandas库的apply函数,结合情感词典计算每条评论的情感得分:
```python
# 计算每个评论的情感得分
def get_sentiment_score(comment):
words = comment.split()
sentiment_score = sum([sentiment_dict.get(word, 0) for word in words])
return sentiment_score
df['sentiment_score'] = df['comment_cut'].apply(get_sentiment_score)
```
其中,'sentiment_score'是新建的一列,用于存储每条评论的情感得分。
6.保存结果。可以使用pandas库的to_excel函数将结果保存到Excel文件中:
```python
# 将结果保存到Excel文件中
df.to_excel('comments_sentiment.xlsx', index=False)
```
其中,'comments_sentiment.xlsx'是保存结果的Excel文件名。
通过以上步骤,就可以使用情感词典对Excel表格中的在线评论进行情感分析了。
阅读全文
相关推荐


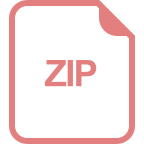
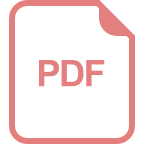
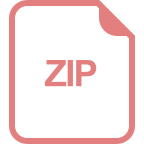
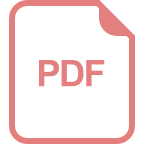
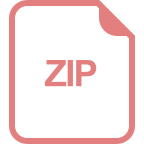






