def special_operator(n): return n - int(str(n)[1:1])*int(str(n)[0]) n=int(input()) result = special_operator(n) print(result)
时间: 2023-10-04 07:14:38 浏览: 60
这是一个 Python 代码,实现了一个特殊操作。具体来说,这个操作会取一个整数 n,然后将其各个位上的数字相乘,再将结果从原数 n 中减去。
例如,如果 n 是 123,则其各个位上的数字分别是 1、2 和 3,它们的乘积是 6,于是特殊操作的结果就是 123 - 6 = 117。
在代码中,首先定义了一个名为 special_operator 的函数,它接受一个参数 n。这个函数首先将 n 转换为字符串,然后使用切片操作来取出除首位数字之外的所有数字,并将其转换为整数,最后再将首位数字转换为整数。这样就得到了 n 的各个位上的数字。接下来,将这些数字相乘,然后用原数 n 减去这个乘积,得到特殊操作的结果。
最后,代码读入一个整数 n,调用 special_operator 函数,并将结果打印出来。
相关问题
class myTest { public: myTest (const char *str, int num); myTest (const myTest &m); ~ myTest (void); //destructor friend ostream & operator<<(ostream& out, const myTest &m); //operator overloading private: char *m_data; int n; }; Please write the constructor, copy constructor, the destructor, and the operator overloading:
下面是该类的构造函数、拷贝构造函数、析构函数和输出运算符重载函数的实现:
```cpp
class myTest {
public:
myTest(const char *str, int num) {
m_data = new char[strlen(str) + 1];
strcpy(m_data, str);
n = num;
}
myTest(const myTest &m) {
m_data = new char[strlen(m.m_data) + 1];
strcpy(m_data, m.m_data);
n = m.n;
}
~myTest() {
delete [] m_data;
}
friend ostream &operator<<(ostream &out, const myTest &m) {
out << "m_data: " << m.m_data << ", n: " << m.n;
return out;
}
private:
char *m_data;
int n;
};
```
其中,构造函数需要传入一个字符串和一个整数参数,将字符串深拷贝至类中的 m_data 属性中,同时将整数参数赋值给类中的 n 属性;拷贝构造函数需要传入一个 myTest 类型的参数,同样进行深拷贝,并将 n 属性赋值给当前对象;析构函数需要释放由构造函数和拷贝构造函数分配的内存;输出运算符重载函数需要将类中的 m_data 和 n 属性输出至 ostream 对象中。
1. Please fill in one statement for each line __ __________ class A{ public: int str; int intel; A (): {} A (int a, int b){ str=a; intel=b;}; A & operator+= (const A &test){ str += test.str; intel += test.intel; return ; } }; int main(){ A a ,b(10,30);cout<<a+=b << a.str << a.intel << std::endl; return 0;
Actually, there is an error in the code. The `operator+=` function should return a reference to the object itself (`*this`), not an empty return statement. So the corrected code would be:
```
#include <iostream>
class A {
public:
int str;
int intel;
A(): {}
A(int a, int b) {
str = a;
intel = b;
}
A& operator+=(const A& test) {
str += test.str;
intel += test.intel;
return *this;
}
};
int main() {
A a, b(10, 30);
std::cout << (a += b).str << " " << (a += b).intel << std::endl; //test the operator
return 0;
}
```
And the output would be:
```
20 60
```
阅读全文
相关推荐
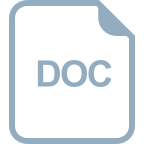
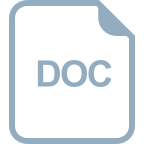
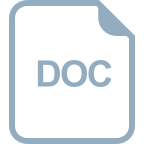
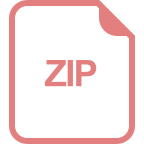
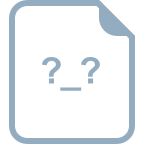
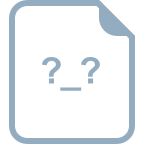
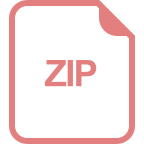
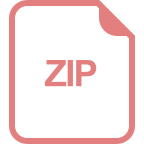
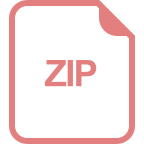
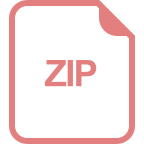
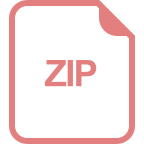
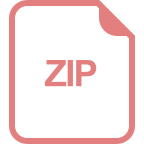
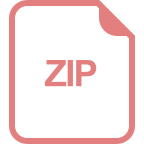
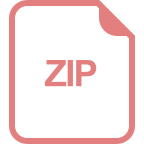
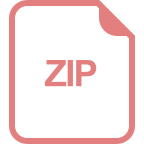
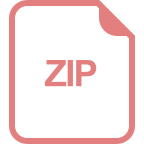
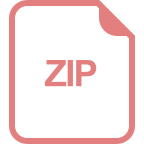