写一段Linux系统上用gcc完成arp报文捕获的代码,代码要求创建原始套接词
时间: 2024-06-12 13:07:16 浏览: 8
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
#include <sys/socket.h>
#include <sys/types.h>
#include <netinet/in.h>
#include <netinet/if_ether.h>
#include <arpa/inet.h>
#include <net/if.h>
#include <unistd.h>
#define BUFFER_LENGTH 65536
int main(int argc, char **argv)
{
int sock_raw;
struct sockaddr_in source, dest;
unsigned char *buffer = (unsigned char *)malloc(BUFFER_LENGTH);
// Create raw socket
sock_raw = socket(AF_PACKET, SOCK_RAW, htons(ETH_P_ARP));
if (sock_raw < 0)
{
printf("Error creating raw socket\n");
return 1;
}
while (1)
{
// Receive ARP packet
memset(buffer, 0, BUFFER_LENGTH);
int len = recvfrom(sock_raw, buffer, BUFFER_LENGTH, 0, NULL, NULL);
if (len < 0)
{
printf("Error receiving packet\n");
return 1;
}
// Parse ARP packet
struct ethhdr *eth = (struct ethhdr *)buffer;
if (ntohs(eth->h_proto) != ETH_P_ARP)
{
printf("Not an ARP packet\n");
continue;
}
struct arphdr *arp = (struct arphdr *)(buffer + sizeof(struct ethhdr));
if (ntohs(arp->ar_op) != ARPOP_REQUEST && ntohs(arp->ar_op) != ARPOP_REPLY)
{
printf("Not an ARP request or reply\n");
continue;
}
// Print ARP packet information
printf("ARP packet:\n");
printf(" Source MAC: %02x:%02x:%02x:%02x:%02x:%02x\n",
eth->h_source[0], eth->h_source[1], eth->h_source[2],
eth->h_source[3], eth->h_source[4], eth->h_source[5]);
printf(" Source IP: %s\n", inet_ntoa(*(struct in_addr *)arp->ar_sip));
printf(" Destination MAC: %02x:%02x:%02x:%02x:%02x:%02x\n",
eth->h_dest[0], eth->h_dest[1], eth->h_dest[2],
eth->h_dest[3], eth->h_dest[4], eth->h_dest[5]);
printf(" Destination IP: %s\n", inet_ntoa(*(struct in_addr *)arp->ar_tip));
}
close(sock_raw);
free(buffer);
return 0;
}
相关推荐
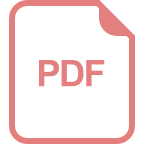














