用jsx实现瀑布流懒加载,写一下
时间: 2024-06-10 10:06:29 浏览: 1
好的,以下是用JSX实现瀑布流懒加载的代码示例:
```jsx
import React, { useState, useEffect, useRef } from 'react';
// 瀑布流容器组件
function WaterfallContainer() {
// 初始瀑布流列数为3
const [columns, setColumns] = useState(3);
// 初始化每一列的高度为0
const [heights, setHeights] = useState(new Array(columns).fill(0));
// 容器的ref
const containerRef = useRef(null);
// 监听窗口大小变化,动态更新瀑布流列数
useEffect(() => {
function handleResize() {
if (containerRef.current) {
const { width } = containerRef.current.getBoundingClientRect();
if (width >= 900) {
setColumns(3);
} else if (width >= 600) {
setColumns(2);
} else {
setColumns(1);
}
}
}
window.addEventListener('resize', handleResize);
return () => window.removeEventListener('resize', handleResize);
}, []);
// 渲染每一项组件
function renderItems() {
// 假设数据源是一个图片数组
const dataSource = [
'https://picsum.photos/id/1003/200/300',
'https://picsum.photos/id/1002/200/300',
'https://picsum.photos/id/1001/200/300',
// ...
];
// 每一项的宽度
const itemWidth = `${100 / columns}%`;
return dataSource.map((item, index) => {
// 找到高度最小的一列
const minHeight = Math.min(...heights);
const minColumnIndex = heights.indexOf(minHeight);
// 更新该列的高度
const newHeights = [...heights];
newHeights[minColumnIndex] += 300; // 假设每一项的高度都是300
setHeights(newHeights);
// 根据该列的高度定位组件
const top = minHeight;
const left = minColumnIndex * (100 / columns);
return (
<div
key={index}
style={{ width: itemWidth, position: 'absolute', top, left }}
>
<img src={item} alt="" />
</div>
);
});
}
// 监听滚动事件,实现懒加载
useEffect(() => {
function handleScroll() {
if (containerRef.current) {
const { scrollTop, scrollHeight, clientHeight } = containerRef.current;
if (scrollHeight - scrollTop === clientHeight) {
// 模拟异步加载数据
setTimeout(() => {
console.log('load more data');
}, 1000);
}
}
}
containerRef.current.addEventListener('scroll', handleScroll);
return () => containerRef.current.removeEventListener('scroll', handleScroll);
}, []);
return (
<div ref={containerRef} style={{ position: 'relative' }}>
{renderItems()}
</div>
);
}
```
相关推荐
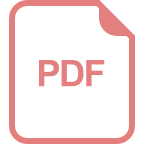
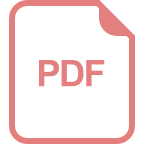
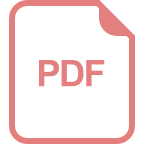
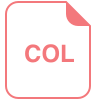
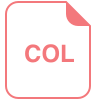
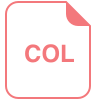
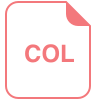
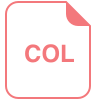









