Linux系统c++中detach用法
时间: 2024-04-06 18:07:53 浏览: 55
在C++中,当我们创建一个线程时,我们可以选择将线程分离(detach)或者等待线程结束(join)。如果我们选择将线程分离,则该线程将在后台运行,而主线程可以继续执行其他任务。如果我们选择等待线程结束,则主线程将阻塞等待,直到子线程执行完毕。
在Linux系统中,我们可以使用`pthread_detach`函数将线程分离。该函数的原型如下:
```c++
#include <pthread.h>
int pthread_detach(pthread_t thread);
```
该函数接受一个线程ID作为参数,并将该线程分离。调用该函数后,该线程的资源将由系统自动回收,而无需使用`pthread_join`等函数等待线程结束。
以下是一个示例代码:
```c++
#include <iostream>
#include <pthread.h>
#include <unistd.h>
using namespace std;
void* thread_func(void* arg)
{
cout << "Thread running..." << endl;
sleep(3);
cout << "Thread exiting..." << endl;
pthread_exit(NULL);
}
int main()
{
pthread_t tid;
int ret = pthread_create(&tid, NULL, thread_func, NULL);
if(ret != 0)
{
cout << "Error: Failed to create thread!" << endl;
return -1;
}
// 分离线程
ret = pthread_detach(tid);
if(ret != 0)
{
cout << "Error: Failed to detach thread!" << endl;
return -1;
}
cout << "Main thread exiting..." << endl;
return 0;
}
```
在该示例中,我们创建了一个线程,并使用`pthread_detach`函数将其分离。主线程继续执行,而子线程在后台运行,最终自动结束并释放资源。
阅读全文
相关推荐
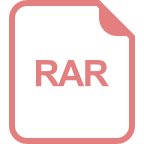
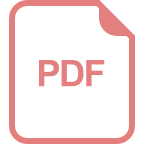
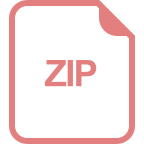
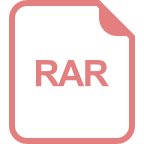
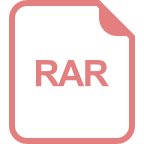
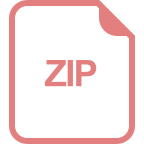
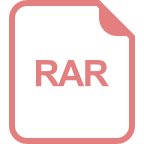
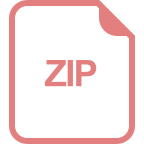
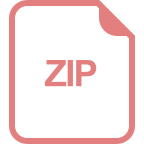
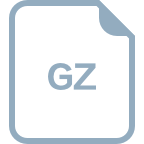
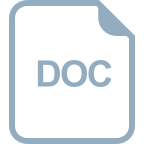
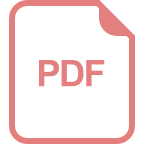
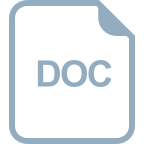
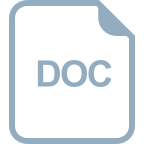
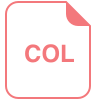
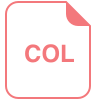
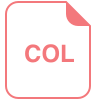

