给定层数,构造满二叉树,之后每次选择一个节点将该节点子树的所有节点全部染红,每次操作后,输出当前二叉树红色节点数量,java实现
时间: 2023-05-19 17:05:14 浏览: 88
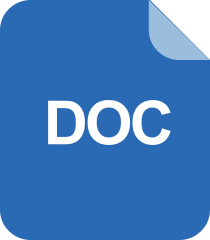
用java实现二叉树的创建和遍历.doc
以下是 Java 实现:
```
import java.util.*;
class TreeNode {
int val;
TreeNode left;
TreeNode right;
boolean isRed;
public TreeNode(int val) {
this.val = val;
this.isRed = false;
}
}
public class RedNodeCounter {
public static void main(String[] args) {
Scanner sc = new Scanner(System.in);
int n = sc.nextInt();
TreeNode root = buildTree(n);
int count = countRedNodes(root);
System.out.println(count);
while (true) {
int val = sc.nextInt();
TreeNode node = findNode(root, val);
if (node == null) {
System.out.println("Node not found!");
continue;
}
paintRed(node);
count = countRedNodes(root);
System.out.println(count);
}
}
public static TreeNode buildTree(int n) {
if (n == 0) {
return null;
}
TreeNode root = new TreeNode(1);
Queue<TreeNode> queue = new LinkedList<>();
queue.offer(root);
int i = 2;
while (i <= n) {
TreeNode node = queue.poll();
node.left = new TreeNode(i++);
node.right = new TreeNode(i++);
queue.offer(node.left);
queue.offer(node.right);
}
return root;
}
public static int countRedNodes(TreeNode root) {
if (root == null) {
return 0;
}
int count = root.isRed ? 1 : 0;
count += countRedNodes(root.left);
count += countRedNodes(root.right);
return count;
}
public static TreeNode findNode(TreeNode root, int val) {
if (root == null) {
return null;
}
if (root.val == val) {
return root;
}
TreeNode left = findNode(root.left, val);
if (left != null) {
return left;
}
TreeNode right = findNode(root.right, val);
if (right != null) {
return right;
}
return null;
}
public static void paintRed(TreeNode node) {
if (node == null) {
return;
}
node.isRed = true;
paintRed(node.left);
paintRed(node.right);
}
}
```
这个程序可以构造给定层数的满二叉树,并支持染红节点和计算红色节点数量的操作。在主函数中,首先构造一棵满二叉树,并输出初始的红色节点数量。然后进入一个循环,每次读入一个节点的值,找到该节点并将其子树染红,然后输出当前的红色节点数量。如果输入的节点不存在,则输出 "Node not found!"。
阅读全文
相关推荐
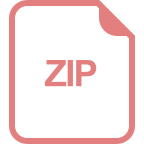
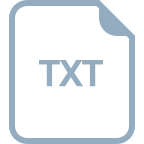
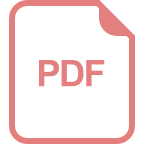
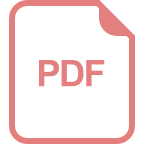
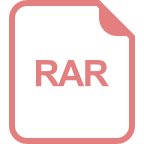
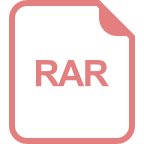
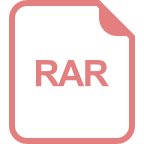
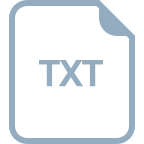
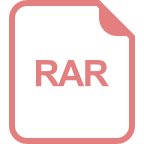
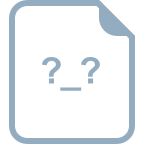
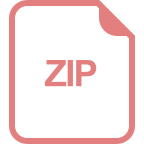
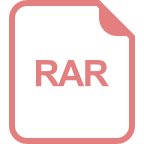