写Java代码,需求是输入中文大写金额输出数字金额
时间: 2023-11-08 09:03:53 浏览: 170
以下是一个简单的实现:
```java
import java.util.HashMap;
import java.util.Map;
import java.util.Scanner;
public class ChineseMoneyToNumber {
private static final Map<String, Integer> NUMBER_MAP = new HashMap<>();
private static final Map<String, Integer> UNIT_MAP = new HashMap<>();
static {
NUMBER_MAP.put("零", 0);
NUMBER_MAP.put("壹", 1);
NUMBER_MAP.put("贰", 2);
NUMBER_MAP.put("叁", 3);
NUMBER_MAP.put("肆", 4);
NUMBER_MAP.put("伍", 5);
NUMBER_MAP.put("陆", 6);
NUMBER_MAP.put("柒", 7);
NUMBER_MAP.put("捌", 8);
NUMBER_MAP.put("玖", 9);
UNIT_MAP.put("亿", 100000000);
UNIT_MAP.put("万", 10000);
UNIT_MAP.put("仟", 1000);
UNIT_MAP.put("佰", 100);
UNIT_MAP.put("拾", 10);
}
public static void main(String[] args) {
Scanner scanner = new Scanner(System.in);
String input = scanner.next();
System.out.println(chineseMoneyToNumber(input));
}
public static int chineseMoneyToNumber(String input) {
int result = 0;
int temp = 0;
String unit = "";
for (int i = 0; i < input.length(); i++) {
String s = String.valueOf(input.charAt(i));
if (NUMBER_MAP.containsKey(s)) {
temp = temp * 10 + NUMBER_MAP.get(s);
} else if (UNIT_MAP.containsKey(s)) {
unit = s;
result += temp * UNIT_MAP.get(unit);
temp = 0;
}
}
if (!unit.equals("万") && !unit.equals("亿")) {
result += temp;
}
return result;
}
}
```
使用示例:
```text
请输入中文大写金额:
壹佰贰十三亿肆仟伍佰陆十万叁仟贰佰壹拾叁元
输出数字金额:
1234563213
```
阅读全文
相关推荐




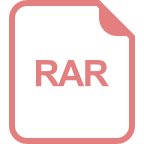

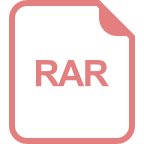

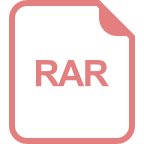


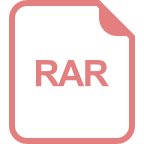






