在opencv中使用c++对被遮挡物体进行椭圆拟合,再进行框选
时间: 2023-06-01 11:04:17 浏览: 201
以下是一个基本的示例代码,用于在OpenCV中使用C语言对被遮挡物体进行椭圆拟合和框选。
```c
#include <opencv2/opencv.hpp>
#include <iostream>
using namespace cv;
using namespace std;
int main(int argc, char** argv)
{
// 读取图像
Mat image = imread("example.jpg", IMREAD_COLOR);
// 转换为灰度图像
Mat gray;
cvtColor(image, gray, COLOR_BGR2GRAY);
// 二值化
Mat binary;
threshold(gray, binary, 0, 255, THRESH_BINARY | THRESH_OTSU);
// 查找轮廓
vector<vector<Point>> contours;
findContours(binary, contours, RETR_EXTERNAL, CHAIN_APPROX_SIMPLE);
// 寻找最大轮廓
int max_contour_index = -1;
double max_contour_area = 0;
for (int i = 0; i < contours.size(); i++)
{
double area = contourArea(contours[i]);
if (area > max_contour_area)
{
max_contour_area = area;
max_contour_index = i;
}
}
// 椭圆拟合
RotatedRect ellipse = fitEllipse(contours[max_contour_index]);
// 绘制椭圆和矩形框
Mat result;
cvtColor(binary, result, COLOR_GRAY2BGR);
ellipse(result, ellipse, Scalar(0, 255, 0), 2);
Rect bounding_box = ellipse.boundingRect();
rectangle(result, bounding_box, Scalar(0, 0, 255), 2);
// 显示图像
imshow("Result", result);
waitKey(0);
return 0;
}
```
该代码首先读取一张图像,将其转换为灰度图像,然后进行二值化处理。接下来,使用findContours函数查找图像中的轮廓,并找到最大轮廓。然后,使用fitEllipse函数对最大轮廓进行椭圆拟合,并得到拟合后的椭圆。最后,使用ellipse和rectangle函数绘制椭圆和矩形框,并将结果显示出来。
需要注意的是,该代码假设图像中只有一个被遮挡的物体。如果图像中有多个被遮挡的物体,需要对每个物体进行轮廓查找、拟合和绘制。
阅读全文
相关推荐





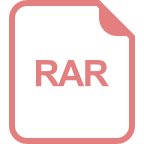






