vue中如何实现在某一区域中多个元素随机定位显示且不重叠给出示例并判断不能重叠
时间: 2024-03-01 08:50:06 浏览: 162
在vue中,可以使用CSS的`position: absolute`和JavaScript的随机数生成来实现在某一区域中多个元素随机定位显示且不重叠。
下面是一个示例代码:
HTML:
```html
<div class="container">
<div class="box" v-for="item in items" :style="{ top: item.top + 'px', left: item.left + 'px' }"></div>
</div>
```
CSS:
```css
.container {
position: relative;
width: 500px;
height: 500px;
}
.box {
position: absolute;
width: 50px;
height: 50px;
background-color: #ccc;
}
```
JavaScript:
```javascript
export default {
data() {
return {
items: []
}
},
mounted() {
this.generateItems()
},
methods: {
generateItems() {
const container = document.querySelector('.container')
const containerWidth = container.clientWidth
const containerHeight = container.clientHeight
for (let i = 0; i < 10; i++) {
const itemWidth = 50
const itemHeight = 50
let left = Math.random() * (containerWidth - itemWidth)
let top = Math.random() * (containerHeight - itemHeight)
while(this.isOverlap(left, top, itemWidth, itemHeight)) {
left = Math.random() * (containerWidth - itemWidth)
top = Math.random() * (containerHeight - itemHeight)
}
this.items.push({
top,
left
})
}
},
isOverlap(left, top, width, height) {
for (const item of this.items) {
if (left < item.left + 50 &&
left + width > item.left &&
top < item.top + 50 &&
top + height > item.top) {
return true
}
}
return false
}
}
}
```
上述代码中,首先在HTML中设定一个容器,然后在CSS中设置容器为`position: relative`,多个元素为`position: absolute`,并且设置宽高和背景颜色。
在JavaScript中,首先使用`Math.random()`生成随机数来计算每个元素的随机位置,并且使用`isOverlap()`方法来判断每个元素是否重叠。如果元素重叠,则重新生成位置。
在mounted生命周期函数中调用`generateItems()`方法来生成元素,并且使用`v-for`指令来渲染每个元素的位置。
这样就可以在某一区域中多个元素随机定位显示且不重叠了。
阅读全文
相关推荐
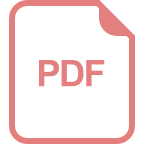
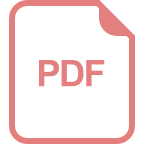
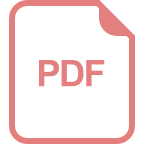










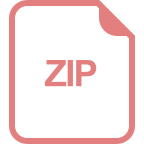