xv6中用户程序在调用了read(0,&a,1) 之后,xv6的反应过程。
时间: 2024-06-07 10:05:52 浏览: 188
在 xv6 中,用户程序可以通过系统调用 `read` 从标准输入中读取数据。当用户程序调用 `read` 时,操作系统会将用户程序阻塞,并等待用户在标准输入中输入数据。如果标准输入中有数据可读,则操作系统会将数据复制到用户程序的缓冲区,并返回实际读取的字节数。如果标准输入中没有数据可读,则操作系统会将用户程序加入到等待输入的进程队列中,并将其阻塞。当用户在标准输入中输入数据后,操作系统会唤醒等待输入的进程,并将数据复制到其缓冲区中,然后返回实际读取的字节数。在 xv6 中,标准输入的文件描述符为 0。因此,当用户程序调用 `read(0, buf, count)` 时,它将从标准输入中读取数据,最多读取 `count` 个字节,并将数据存储到 `buf` 缓冲区中。
相关问题
xv6编写添加系统调用
在 xv6 中添加系统调用需要进行以下步骤:
1. 在 `syscall.h` 文件中添加系统调用号。具体来说,你需要在 `SYS_hoge` 列表中添加一个新的系统调用号,其中 `hoge` 是你的系统调用的名称。例如,如果你的系统调用名称是 `mycall`,则可以在 `syscall.h` 文件中添加以下代码:
```
#define SYS_mycall 22
```
2. 在 `syscall.c` 文件中添加系统调用的具体实现。具体来说,你需要在 `syscalls[]` 数组中添加一个新的结构体,其中包含你的系统调用的名称和具体的实现函数。例如,如果你的系统调用名称是 `mycall`,则可以在 `syscall.c` 文件中添加以下代码:
```
static int
sys_mycall(void)
{
// 在这里编写你的系统调用的具体实现
return 0;
}
static struct {
int nargs;
int (*func)(void);
} syscalls[] = {
{ 0, sys_fork },
{ 1, sys_exit },
{ 0, sys_wait },
{ 1, sys_pipe },
{ 4, sys_read },
{ 4, sys_write },
{ 2, sys_close },
{ 1, sys_mycall }, // 添加新的系统调用
};
```
3. 在 `user.h` 文件中添加用户空间的系统调用函数声明。具体来说,你需要添加一个新的函数声明,其中包含你的系统调用的名称和参数。例如,如果你的系统调用名称是 `mycall`,则可以在 `user.h` 文件中添加以下代码:
```
int mycall(void);
```
4. 在用户程序中调用你的系统调用。具体来说,你可以在用户程序中调用你的系统调用函数,例如:
```
#include "user.h"
int
main(int argc, char *argv[])
{
mycall(); // 调用你的系统调用
exit();
}
```
5. 在内核态的系统调用函数中添加系统调用的具体实现。具体来说,你需要在 `syscall()` 函数中添加一个新的 `case` 分支,其中包含你的系统调用的具体实现。例如,如果你的系统调用名称是 `mycall`,则可以在 `syscall()` 函数中添加以下代码:
```
case SYS_mycall:
argint(0, &arg1);
// 在这里调用你的系统调用的具体实现
return 0;
```
6. 最后,重新编译 xv6 并运行你的用户程序。在 xv6 的命令行下输入你的程序名称即可运行。例如,如果你的用户程序名称是 `myprog`,则可以通过输入 `myprog` 命令来运行它,其中包含了你的系统调用的调用。
xv6编写添加用户程序如何具体实现
在xv6中添加用户需要进行以下步骤:
1. 修改user.h头文件,添加新用户的定义,包括用户名和密码。
2. 修改usertests.c文件,增加测试新用户的代码。
3. 修改userinit.c文件,添加新用户的代码。具体来说,需要在init_user()函数中调用mkuser函数创建新用户,并将用户信息写入到文件系统中。
4. 修改Makefile文件,将新的源文件添加到编译选项中。
下面是一些具体的实现细节:
1. 在user.h中添加新用户的定义,例如:
```
#define MAX_USERS 10
struct user {
char name[16];
char password[16];
};
extern struct user users[MAX_USERS];
```
2. 在usertests.c中增加测试新用户的代码,例如:
```
void
test_add_user(void)
{
// Add a new user
int result = adduser("testuser", "testpass");
assert(result == 0);
// Try to login as the new user
result = login("testuser", "testpass");
assert(result == 0);
printf("adduser test passed\n");
}
```
3. 在userinit.c中添加新用户的代码,例如:
```
void
init_users(void)
{
// Create a new user
mkuser("testuser", "testpass");
// Create other users...
}
void
mkuser(char *name, char *password)
{
struct user u;
// Check if user already exists
if (getuser(name, &u) == 0) {
printf("User %s already exists\n", name);
return;
}
// Find an empty slot for the new user
int i;
for (i = 0; i < MAX_USERS; i++) {
if (users[i].name[0] == 0) {
strncpy(users[i].name, name, sizeof(users[i].name));
strncpy(users[i].password, password, sizeof(users[i].password));
// Write user info to file system
write_user(&users[i]);
return;
}
}
printf("Cannot create user %s, too many users\n", name);
}
int
getuser(char *name, struct user *u)
{
int fd = open("users", O_RDONLY);
if (fd < 0)
return -1;
struct user temp;
while (read(fd, &temp, sizeof(temp)) == sizeof(temp)) {
if (strcmp(temp.name, name) == 0) {
close(fd);
memcpy(u, &temp, sizeof(temp));
return 0;
}
}
close(fd);
return -1;
}
void
write_user(struct user *u)
{
int fd = open("users", O_WRONLY | O_APPEND | O_CREAT, 0666);
if (fd < 0)
return;
write(fd, u, sizeof(*u));
close(fd);
}
```
4. 在Makefile中添加新的源文件,例如:
```
UPROGS=\
_cat\
_echo\
_forktest\
_grep\
_init\
_kill\
_ln\
_ls\
_mkdir\
_rm\
_sh\
_stressfs\
_usertests\
_wc\
_adduser\
_login\
_logout\
```
通过以上步骤,就可以在xv6中添加新用户了。
阅读全文
相关推荐
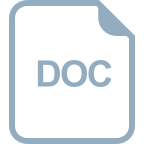
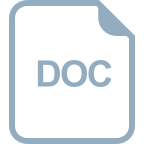
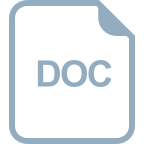
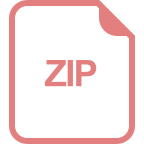
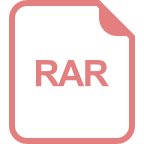
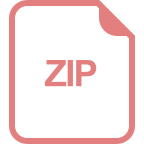
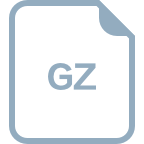
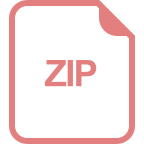
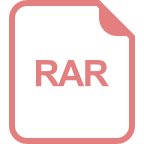
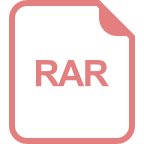
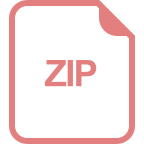
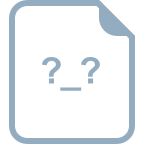
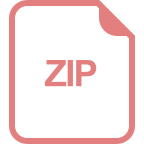
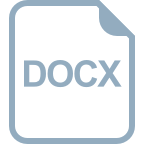
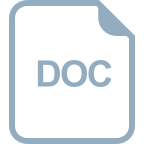
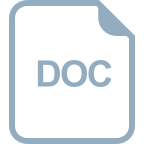
