LINUX双线程交替打印奇偶数
时间: 2023-08-04 08:08:32 浏览: 87
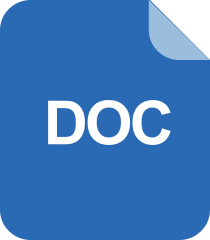
linux下C语言多线程编程实例
好的,这是一个经典的多线程问题,可以使用线程同步的方式解决。具体实现如下:
```c
#include <stdio.h>
#include <pthread.h>
pthread_mutex_t mutex;
pthread_cond_t cond;
int count = 1;
void *print_odd(void *arg)
{
while (count <= 10)
{
pthread_mutex_lock(&mutex);
if (count % 2 != 0)
{
printf("Thread 1: %d\n", count++);
pthread_cond_signal(&cond);
}
else
{
pthread_cond_wait(&cond, &mutex);
}
pthread_mutex_unlock(&mutex);
}
return NULL;
}
void *print_even(void *arg)
{
while (count <= 10)
{
pthread_mutex_lock(&mutex);
if (count % 2 == 0)
{
printf("Thread 2: %d\n", count++);
pthread_cond_signal(&cond);
}
else
{
pthread_cond_wait(&cond, &mutex);
}
pthread_mutex_unlock(&mutex);
}
return NULL;
}
int main()
{
pthread_t tid1, tid2;
pthread_mutex_init(&mutex, NULL);
pthread_cond_init(&cond, NULL);
pthread_create(&tid1, NULL, print_odd, NULL);
pthread_create(&tid2, NULL, print_even, NULL);
pthread_join(tid1, NULL);
pthread_join(tid2, NULL);
pthread_mutex_destroy(&mutex);
pthread_cond_destroy(&cond);
return 0;
}
```
这段代码中,我们使用了一个互斥锁和条件变量,其中互斥锁用于保护共享变量 count 的访问,条件变量用于线程之间的通信。两个线程分别打印奇数和偶数,通过对 count 取模的方式来确定哪个线程应该打印数值。如果当前不是该线程打印的数值,就等待条件变量的信号,否则就打印数值并发送信号给另一个线程。注意,线程之间的通信必须在互斥锁的保护下进行,以避免竞争条件的发生。
阅读全文
相关推荐
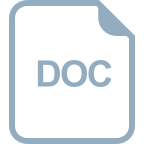
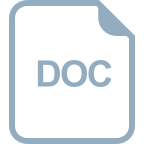
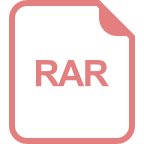
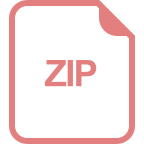
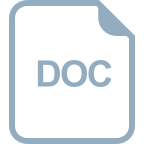
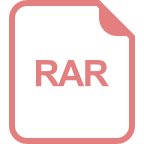
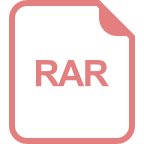
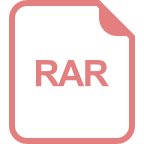
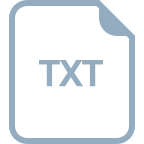
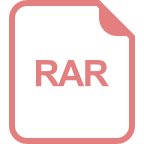
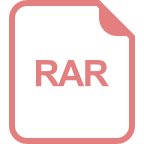
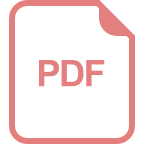