php定义学生类使用
时间: 2023-07-15 11:15:37 浏览: 58
以下是一个简单的 PHP 学生类的定义示例:
```php
class Student {
// 定义属性
public $name;
public $age;
public $grade;
// 定义方法
public function display() {
echo "Name: " . $this->name . "<br>";
echo "Age: " . $this->age . "<br>";
echo "Grade: " . $this->grade . "<br>";
}
}
```
在上面的代码中,`Student` 类具有三个属性:`name`、`age` 和 `grade`。它还具有一个 `display()` 方法,用于显示学生的详细信息。
要创建一个 `Student` 对象并设置其属性,可以使用以下代码:
```php
// 创建一个新的 Student 对象
$student = new Student();
// 设置属性
$student->name = "John";
$student->age = 18;
$student->grade = "Senior";
// 调用 display() 方法以显示学生信息
$student->display();
```
输出结果应该类似于:
```
Name: John
Age: 18
Grade: Senior
```
相关问题
php定义学生类,计算平均分
以下是 PHP 中定义一个学生类,并计算平均分的示例代码:
```php
class Student {
private $name;
private $scores = array();
public function __construct($name, $scores) {
$this->name = $name;
$this->scores = $scores;
}
public function getAverageScore() {
$sum = 0;
foreach($this->scores as $score) {
$sum += $score;
}
return $sum / count($this->scores);
}
}
$student1 = new Student("Alice", array(85, 90, 95));
$averageScore1 = $student1->getAverageScore();
echo "Student " . $student1->getName() . " has an average score of " . $averageScore1;
$student2 = new Student("Bob", array(75, 80, 85, 90));
$averageScore2 = $student2->getAverageScore();
echo "Student " . $student2->getName() . " has an average score of " . $averageScore2;
```
在上面的示例中,我们首先定义了一个 `Student` 类,该类有两个属性:`name` 和 `scores`。`name` 属性存储学生的姓名,`scores` 属性存储学生的分数数组。
我们还定义了一个构造函数 `__construct`,它接受两个参数:学生的姓名和分数数组,并将它们存储在 `$name` 和 `$scores` 属性中。
最后,我们还定义了一个 `getAverageScore` 方法,该方法计算学生的平均分数,并将其返回。
在主程序中,我们创建了两个学生对象,分别为 `student1` 和 `student2`。我们调用 `getAverageScore` 方法来计算每个学生的平均分,并将结果输出到屏幕上。
php定义一个学生类_定义person类及其子类,并按要求设计、定义和实例化类 (实例)...
好的,我来帮您解答这个问题。
首先,我们来定义一个 `Person` 类,它包含 `name` 和 `age` 两个属性,以及 `sayHello` 方法:
```php
class Person {
public $name;
public $age;
public function sayHello() {
echo "Hello, my name is {$this->name} and I am {$this->age} years old.\n";
}
}
```
接下来,我们来定义 `Student` 类,它继承自 `Person` 类,并增加一个 `major` 属性和一个 `study` 方法:
```php
class Student extends Person {
public $major;
public function study() {
echo "{$this->name} is studying {$this->major}.\n";
}
}
```
现在,我们已经定义了 `Person` 和 `Student` 两个类,接下来我们可以实例化它们:
```php
// 创建一个 Person 对象
$person = new Person();
$person->name = "Alice";
$person->age = 20;
$person->sayHello(); // 输出:Hello, my name is Alice and I am 20 years old.
// 创建一个 Student 对象
$student = new Student();
$student->name = "Bob";
$student->age = 18;
$student->major = "Computer Science";
$student->sayHello(); // 输出:Hello, my name is Bob and I am 18 years old.
$student->study(); // 输出:Bob is studying Computer Science.
```
以上就是一个简单的示例,展示了如何定义 `Person` 和 `Student` 两个类,并实例化它们。
相关推荐
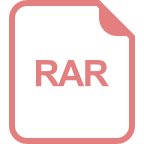
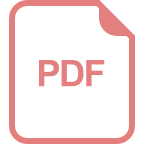
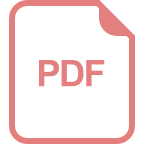












