用spring boot编写一个个人博客系统中的用户互评功能
时间: 2024-05-01 18:19:41 浏览: 105
首先,我们需要定义一个用于存储用户互评的数据表,可以在MySQL数据库中创建一个名为`user_comment`的表,包含以下字段:
- `id`:主键,自增长
- `from_user_id`:评论发起用户的ID
- `to_user_id`:被评论用户的ID
- `comment`:评论内容
- `create_time`:评论时间
接下来,我们可以使用Spring Boot来实现用户互评功能,具体步骤如下:
1. 定义实体类
定义`UserComment`实体类,用于映射`user_comment`数据表中的字段。
```java
@Entity
@Table(name = "user_comment")
public class UserComment {
@Id
@GeneratedValue(strategy = GenerationType.IDENTITY)
private Long id;
@Column(name = "from_user_id")
private Long fromUserId;
@Column(name = "to_user_id")
private Long toUserId;
private String comment;
@Column(name = "create_time")
private Date createTime;
// 省略getter和setter方法
}
```
2. 定义数据访问层
定义`UserCommentRepository`接口,继承`JpaRepository`,用于对`user_comment`表进行增删改查操作。
```java
public interface UserCommentRepository extends JpaRepository<UserComment, Long> {
List<UserComment> findByToUserId(Long toUserId);
}
```
3. 定义服务层
定义`UserCommentService`服务类,用于对用户互评功能进行业务逻辑处理。
```java
@Service
public class UserCommentService {
@Autowired
private UserCommentRepository userCommentRepository;
public void addComment(UserComment userComment) {
userComment.setCreateTime(new Date());
userCommentRepository.save(userComment);
}
public List<UserComment> getComments(Long userId) {
return userCommentRepository.findByToUserId(userId);
}
}
```
4. 定义控制器
定义`UserCommentController`控制器类,用于接收前端请求,并调用服务层中的方法进行业务逻辑处理。
```java
@RestController
@RequestMapping("/comment")
public class UserCommentController {
@Autowired
private UserCommentService userCommentService;
@PostMapping("/add")
public void addComment(@RequestBody UserComment userComment) {
userCommentService.addComment(userComment);
}
@GetMapping("/get")
public List<UserComment> getComments(Long userId) {
return userCommentService.getComments(userId);
}
}
```
至此,我们已经完成了用户互评功能的实现。在前端页面中,可以通过AJAX调用`/comment/add`接口来提交评论,通过`/comment/get`接口来获取用户收到的评论列表。
阅读全文
相关推荐
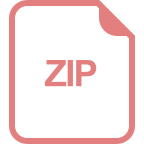
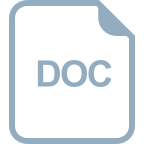
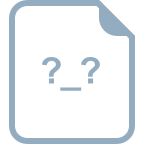
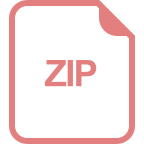
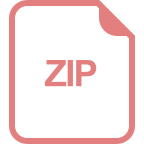
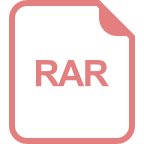
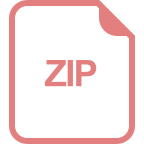
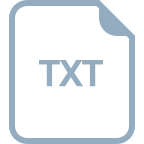
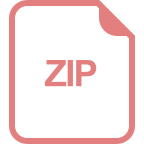
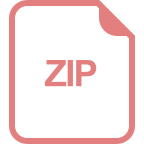
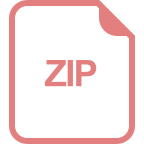
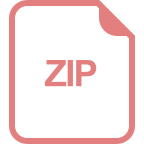
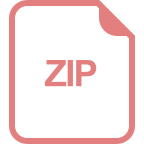
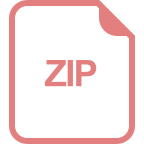
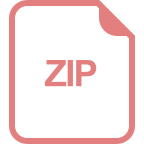
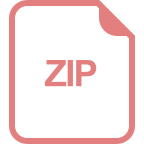