Tkinter编写程序动态演示钟摆的摆动
时间: 2024-01-03 17:05:56 浏览: 32
下面是一个使用Tkinter编写的程序,可以动态演示钟摆的摆动。
```python
import tkinter as tk
import math
class Pendulum:
def __init__(self, canvas, length, angle, mass):
self.canvas = canvas
self.length = length
self.mass = mass
self.angle = angle
self.velocity = 0
self.acceleration = 0
self.create()
def create(self):
x = self.canvas.winfo_width() / 2
y = self.canvas.winfo_height() / 2
self.anchor = self.canvas.create_oval(x-5, y-5, x+5, y+5, fill="black")
self.body = self.canvas.create_line(x, y, x+self.length*math.sin(self.angle), y+self.length*math.cos(self.angle), width=self.mass, capstyle="round")
def update(self, dt):
g = 9.8
self.acceleration = -g/self.length*math.sin(self.angle)
self.velocity += self.acceleration*dt
self.angle += self.velocity*dt
x = self.canvas.winfo_width() / 2
y = self.canvas.winfo_height() / 2
self.canvas.coords(self.body, x, y, x+self.length*math.sin(self.angle), y+self.length*math.cos(self.angle))
class App:
def __init__(self, master):
self.master = master
self.canvas = tk.Canvas(master, width=600, height=600, bg="white")
self.canvas.pack(fill=tk.BOTH, expand=True)
self.pendulum = Pendulum(self.canvas, 200, math.pi/4, 20)
self.canvas.bind("<Configure>", self.on_resize)
self.update()
def on_resize(self, event):
self.canvas.delete("all")
self.canvas.config(width=event.width, height=event.height)
self.pendulum.create()
def update(self):
dt = 0.01
self.pendulum.update(dt)
self.master.after(int(dt*1000), self.update)
root = tk.Tk()
app = App(root)
root.mainloop()
```
运行程序后,会出现一个窗口,里面显示了一个钟摆。钟摆的长度、角度和质量可以在程序中进行调整。
程序中使用了Tkinter的Canvas组件来绘制钟摆。首先创建了一个Pendulum类来表示钟摆,其中包含了钟摆的长度、角度、质量、速度和加速度等属性。在Pendulum类的create方法中,使用Canvas组件的create_oval方法创建了钟摆的锚点,使用create_line方法创建了钟摆的主体。在update方法中,根据物理公式计算了钟摆的加速度和速度,然后更新了钟摆的角度,并使用Canvas组件的coords方法更新了钟摆的主体。在App类中,使用Canvas组件创建了一个窗口,并创建了一个Pendulum对象,然后在update方法中循环调用Pendulum对象的update方法,以更新钟摆的状态。
希望这个程序能够帮助到你。
相关推荐
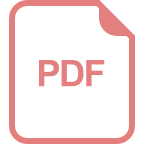
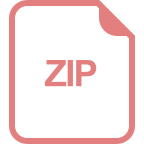














