java excel文件批量导入数据 并生成校验失败数据的excel文件
时间: 2023-12-26 09:06:51 浏览: 132
可以使用Apache POI库来实现Java中的Excel文件操作。以下是一个简单的代码示例,可以批量导入数据并生成校验失败数据的Excel文件:
```java
import java.io.FileInputStream;
import java.io.FileOutputStream;
import java.io.IOException;
import java.util.ArrayList;
import java.util.Iterator;
import java.util.List;
import org.apache.poi.ss.usermodel.Cell;
import org.apache.poi.ss.usermodel.Row;
import org.apache.poi.ss.usermodel.Sheet;
import org.apache.poi.ss.usermodel.Workbook;
import org.apache.poi.xssf.usermodel.XSSFWorkbook;
public class ExcelImport {
public static void main(String[] args) throws IOException {
// 读取Excel文件
FileInputStream inputStream = new FileInputStream("input.xlsx");
Workbook workbook = new XSSFWorkbook(inputStream);
Sheet sheet = workbook.getSheetAt(0);
// 遍历Excel文件中的数据
Iterator<Row> iterator = sheet.iterator();
List<String[]> validData = new ArrayList<>(); // 保存校验通过的数据
List<String[]> invalidData = new ArrayList<>(); // 保存校验失败的数据
while (iterator.hasNext()) {
Row currentRow = iterator.next();
Iterator<Cell> cellIterator = currentRow.iterator();
String[] rowData = new String[currentRow.getLastCellNum()];
boolean valid = true; // 校验标识
while (cellIterator.hasNext()) {
Cell currentCell = cellIterator.next();
int columnIndex = currentCell.getColumnIndex();
rowData[columnIndex] = currentCell.getStringCellValue();
// 进行校验,如果校验失败,设置valid为false
// ...
}
if (valid) {
validData.add(rowData);
} else {
invalidData.add(rowData);
}
}
// 生成校验失败的数据的Excel文件
Workbook outputWorkbook = new XSSFWorkbook();
Sheet outputSheet = outputWorkbook.createSheet("Invalid Data");
for (int i = 0; i < invalidData.size(); i++) {
Row row = outputSheet.createRow(i);
String[] rowData = invalidData.get(i);
for (int j = 0; j < rowData.length; j++) {
Cell cell = row.createCell(j);
cell.setCellValue(rowData[j]);
}
}
FileOutputStream outputStream = new FileOutputStream("output.xlsx");
outputWorkbook.write(outputStream);
outputWorkbook.close();
// 关闭文件流
workbook.close();
inputStream.close();
outputStream.close();
}
}
```
在上面的代码中,我们首先读取了一个名为“input.xlsx”的Excel文件,并遍历其中的所有行和单元格。在遍历过程中,我们可以进行数据校验,并将校验通过和校验失败的数据分别保存到`validData`和`invalidData`列表中。
接下来,我们创建了一个名为“Invalid Data”的新工作表,并将校验失败的数据写入其中。最后,我们将新的Excel文件写入名为“output.xlsx”的文件中,并关闭所有文件流。
请注意,上面的代码示例仅供参考。具体实现方式可能因为实际业务需求而有所不同。
阅读全文
相关推荐












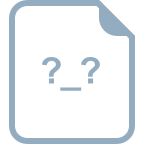
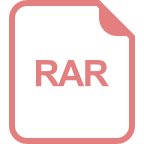




