c++ QT Opencv 实现对raw文件长宽深度信息的获取方法
时间: 2024-03-08 16:50:27 浏览: 23
可以使用 C++ 编程语言结合 Qt 和 OpenCV 库来实现对 raw 文件长、宽、深度信息的获取。以下是一个简单的代码示例:
```c++
#include <iostream>
#include <fstream>
#include <opencv2/opencv.hpp>
#include <QCoreApplication>
using namespace std;
using namespace cv;
const int WIDTH = 512; // 宽度
const int HEIGHT = 512; // 高度
const int DEPTH = 40; // 深度
int main(int argc, char *argv[]) {
QCoreApplication a(argc, argv);
// 读取数据
ifstream fin("data.raw", ios::binary); // 打开二进制文件
if (!fin) {
cerr << "Failed to open file!" << endl;
return 1;
}
unsigned short data[WIDTH][HEIGHT][DEPTH];
fin.read(reinterpret_cast<char*>(&data[0][0][0]), WIDTH * HEIGHT * DEPTH * sizeof(unsigned short));
fin.close(); // 关闭文件
// 转换为 OpenCV Mat 格式
Mat volume(DEPTH, HEIGHT, WIDTH, CV_16UC1, reinterpret_cast<void*>(&data[0][0][0]));
// 输出信息
cout << "Width: " << volume.size[2] << endl; // 宽度
cout << "Height: " << volume.size[1] << endl; // 高度
cout << "Depth: " << volume.size[0] << endl; // 深度
return a.exec();
}
```
这段代码假设了数据类型为无符号 16 位整型,数据尺寸为 512x512x40,数据存储顺序为行优先。首先打开文件,然后使用 ifstream 类的 read 函数读取数据。接着使用 OpenCV 中的 Mat 类来将数据转换为 OpenCV Mat 格式。最后输出长、宽、深度等信息。
这段代码需要在 Qt 项目中使用,需要将 OpenCV 库和相关头文件添加到项目中,并使用 QCoreApplication 类来运行程序。
相关推荐
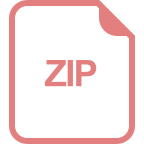
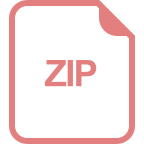
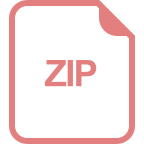














