stm32oled实时显示时间,使用rtc模块,用stm32标准库,请帮我编写出来
时间: 2023-10-16 10:06:18 浏览: 39
好的,以下是一个简单的示例代码,可以实现使用RTC模块和OLED显示器在STM32上显示实时时间:
```c
#include "stm32f10x.h"
#include "stm32f10x_rcc.h"
#include "stm32f10x_gpio.h"
#include "stm32f10x_i2c.h"
#include "stm32f10x_rtc.h"
#include "stm32f10x_pwr.h"
#include "stm32f10x_bkp.h"
#include "stm32f10x_usart.h"
#include "oled.h"
#include <stdio.h>
RTC_InitTypeDef RTC_InitStructure;
RTC_TimeTypeDef RTC_TimeStructure;
RTC_DateTypeDef RTC_DateStructure;
void RTC_Configuration(void)
{
RCC_APB1PeriphClockCmd(RCC_APB1Periph_PWR | RCC_APB1Periph_BKP, ENABLE);
PWR_BackupAccessCmd(ENABLE);
BKP_DeInit();
RCC_LSEConfig(RCC_LSE_ON);
while (RCC_GetFlagStatus(RCC_FLAG_LSERDY) == RESET);
RCC_RTCCLKConfig(RCC_RTCCLKSource_LSE);
RCC_RTCCLKCmd(ENABLE);
RTC_InitStructure.RTC_AsynchPrediv = 0x7F;
RTC_InitStructure.RTC_SynchPrediv = 0x00FF;
RTC_InitStructure.RTC_HourFormat = RTC_HourFormat_24;
RTC_Init(&RTC_InitStructure);
}
void RTC_GetTimeDate(void)
{
RTC_GetTime(RTC_Format_BIN, &RTC_TimeStructure);
RTC_GetDate(RTC_Format_BIN, &RTC_DateStructure);
}
void OLED_ShowTimeDate(void)
{
char buffer[16];
sprintf(buffer, "%02d:%02d:%02d", RTC_TimeStructure.RTC_Hours, RTC_TimeStructure.RTC_Minutes, RTC_TimeStructure.RTC_Seconds);
OLED_ShowString(0,0,(uint8_t*)buffer);
sprintf(buffer, "%04d-%02d-%02d", RTC_DateStructure.RTC_Year+2000, RTC_DateStructure.RTC_Month, RTC_DateStructure.RTC_Date);
OLED_ShowString(0,16,(uint8_t*)buffer);
}
int main(void)
{
RCC_APB2PeriphClockCmd(RCC_APB2Periph_GPIOA | RCC_APB2Periph_GPIOB | RCC_APB2Periph_AFIO, ENABLE);
RCC_APB1PeriphClockCmd(RCC_APB1Periph_I2C1, ENABLE);
RCC_APB1PeriphClockCmd(RCC_APB1Periph_USART2, ENABLE);
RCC_AHBPeriphClockCmd(RCC_AHBPeriph_DMA1, ENABLE);
GPIO_PinRemapConfig(GPIO_Remap_USART2, ENABLE);
OLED_Init();
RTC_Configuration();
while(1)
{
RTC_GetTimeDate();
OLED_ShowTimeDate();
Delay_ms(1000);
}
}
```
这个示例代码使用了STM32标准库函数来配置RTC模块,并使用OLED显示器来显示实时时间和日期。注意,这里使用了一个名为"OLED"的库,您需要自己实现该库来驱动OLED屏幕。此外,您需要自己编写一个名为"Delay_ms"的延时函数来实现1秒钟的延时。
相关推荐
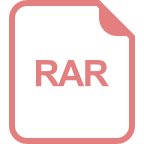
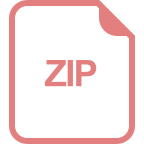














