上面这段程序发生死锁了
时间: 2023-07-19 19:12:30 浏览: 206
你说的没错,这段程序有死锁的问题。在使用缓冲通道时,如果缓冲区已满,写入通道的 Goroutine 会被阻塞,等待其他 Goroutine 读取缓冲区中的数据。但是,在本示例中,第一个 Goroutine 写入 ch1 时,如果 ch1 已满,那么它会被阻塞,等待从 ch1 中读取数据的 Goroutine 取出数据。但是,没有其他 Goroutine 会从 ch1 中读取数据,因为第二个 Goroutine 只会从 ch2 中读取数据。这就导致了死锁。
为了解决这个问题,我们可以使用一个单独的 Goroutine 来协调两个 Goroutine 之间的通信,例如:
```
package main
import (
"fmt"
"sync"
)
func main() {
var wg sync.WaitGroup
wg.Add(3)
ch1 := make(chan int, 1)
ch2 := make(chan int, 1)
go func() {
defer wg.Done()
for i := 1; i <= 100; i += 2 {
fmt.Println("Goroutine 1:", i)
ch1 <- i
<-ch2
}
}()
go func() {
defer wg.Done()
for i := 2; i <= 100; i += 2 {
fmt.Println("Goroutine 2:", i)
ch2 <- i
<-ch1
}
}()
go func() {
defer wg.Done()
for i := 1; i <= 100; i++ {
select {
case num := <-ch1:
fmt.Println("Goroutine 1:", num)
case num := <-ch2:
fmt.Println("Goroutine 2:", num)
}
}
}()
wg.Wait()
}
```
在这个示例中,我们使用了一个额外的 Goroutine,使用 ch1 和 ch2 之间的读写来协调两个 Goroutine 之间的通信。具体来说,第一个 Goroutine 写入 ch1,等待从 ch2 中读取数据,第二个 Goroutine 写入 ch2,等待从 ch1 中读取数据。这样,就可以确保 ch1 和 ch2 中的数据都被正确地读取和写入,避免了死锁的问题。
阅读全文
相关推荐
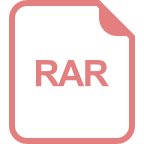
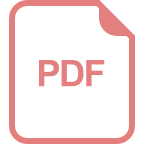
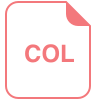
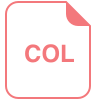
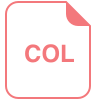
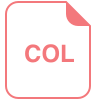
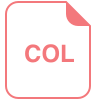
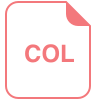
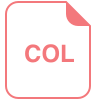
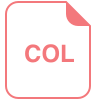
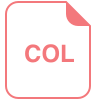
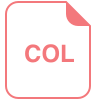
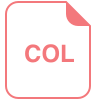
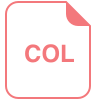
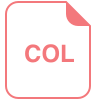
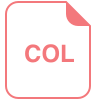
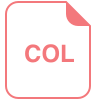