c# 通过反射获取静态类成员
时间: 2023-11-22 08:59:42 浏览: 86
可以使用 `System.Reflection` 命名空间中的 `Type` 类来获取静态类成员。下面是一个示例代码:
```csharp
using System;
using System.Reflection;
public static class MyStaticClass
{
public static int MyStaticField = 42;
public static void MyStaticMethod()
{
Console.WriteLine("Hello from MyStaticMethod!");
}
}
class Program
{
static void Main(string[] args)
{
// 获取 MyStaticClass 类型
Type myStaticClassType = typeof(MyStaticClass);
// 获取 MyStaticField 字段
FieldInfo myStaticFieldInfo = myStaticClassType.GetField("MyStaticField");
// 获取 MyStaticMethod 方法
MethodInfo myStaticMethodInfo = myStaticClassType.GetMethod("MyStaticMethod");
// 输出 MyStaticField 的值
int myStaticFieldValue = (int)myStaticFieldInfo.GetValue(null);
Console.WriteLine("MyStaticField = {0}", myStaticFieldValue);
// 调用 MyStaticMethod 方法
myStaticMethodInfo.Invoke(null, null);
}
}
```
在上面的示例中,我们使用 `typeof(MyStaticClass)` 来获取 `MyStaticClass` 类型,然后使用 `GetField` 方法获取 `MyStaticField` 字段的 `FieldInfo` 对象,并使用 `GetValue` 方法获取该字段的值。然后,我们使用 `GetMethod` 方法获取 `MyStaticMethod` 方法的 `MethodInfo` 对象,并使用 `Invoke` 方法调用该方法。注意,由于 `MyStaticMethod` 是静态方法,因此我们将第一个参数传递为 `null`。
相关推荐
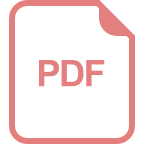
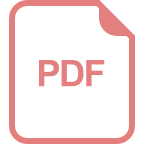
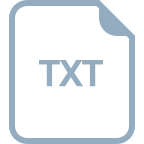














