如何将Byte*图像用二进制的方式保存为图像
时间: 2024-05-14 12:12:10 浏览: 22
1. 将Byte*图像转换为二进制数据:
```c++
unsigned char* imageData = // Byte*图像数据
int imageSize = // 图像数据的大小
std::vector<unsigned char> binaryData(imageSize * 8);
for (int i = 0; i < imageSize; i++) {
for (int j = 0; j < 8; j++) {
binaryData[i * 8 + j] = (imageData[i] >> j) & 1;
}
}
```
2. 将二进制数据保存为图像文件:
```c++
int width = // 图像的宽度
int height = // 图像的高度
std::ofstream outFile("binary_image.bmp", std::ios::binary);
if (outFile) {
// BMP文件头
char bmpFileHeader[14] = { 'B', 'M', 0, 0, 0, 0, 0, 0, 0, 0, 54, 0, 0, 0 };
// BMP信息头
char bmpInfoHeader[40] = { 40, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0,
1, 0, 1, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0 };
// 计算图像数据的大小
int imageDataSize = ((width * height * 1 + 31) / 32) * 4;
// BMP文件头中的文件大小
*(int*)&bmpFileHeader[2] = 54 + imageDataSize;
// BMP信息头中的图像宽度
*(int*)&bmpInfoHeader[4] = width;
// BMP信息头中的图像高度
*(int*)&bmpInfoHeader[8] = height;
// BMP信息头中的图像数据大小
*(int*)&bmpInfoHeader[20] = imageDataSize;
// 写入BMP文件头和BMP信息头
outFile.write(bmpFileHeader, 14);
outFile.write(bmpInfoHeader, 40);
// 写入图像数据
for (int i = height - 1; i >= 0; i--) {
for (int j = 0; j < width; j++) {
unsigned char pixel = 255 * binaryData[i * width + j];
outFile.write((char*)&pixel, 1);
}
// 每行的字节数必须是4的倍数
int paddingSize = ((width * 1 + 3) / 4) * 4 - width;
if (paddingSize > 0) {
char padding[3] = { 0, 0, 0 };
outFile.write(padding, paddingSize);
}
}
outFile.close();
}
```
保存的图像文件是黑白图像,每个像素只有一个二进制值,它表示原始图像中的一个字节的每个位的值。其中,值为1的像素是白色的,值为0的像素是黑色的。
相关推荐
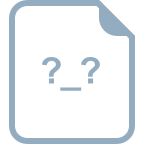














