C++逗号分割字符
时间: 2023-07-11 10:03:47 浏览: 57
在 C++ 中,可以使用字符串流 stringstream 和 getline 函数来实现逗号分割字符。下面是一个示例代码:
```c++
#include <iostream>
#include <string>
#include <sstream>
#include <vector>
using namespace std;
int main() {
string input = "a,b,c,d,e";
stringstream ss(input);
string token;
vector<string> tokens;
while (getline(ss, token, ',')) {
tokens.push_back(token);
}
for (auto t : tokens) {
cout << t << endl;
}
return 0;
}
```
输出结果为:
```
a
b
c
d
e
```
在这个例子中,我们将输入字符串 `a,b,c,d,e` 放入 stringstream 对象中,然后使用 getline 函数和逗号作为分隔符来获取每个子字符串,并将它们存储在 vector 容器中。最后,我们用一个 for 循环遍历 vector 容器并输出每个子字符串。
相关问题
c++逗号分割字符串
在C++中,可以使用stringstream和getline函数来逗号分割字符串。以下是示例代码:
```c++
#include <iostream>
#include <sstream>
#include <string>
#include <vector>
using namespace std;
int main() {
string str = "apple,banana,orange,grape";
vector<string> tokens;
stringstream ss(str);
string token;
while (getline(ss, token, ',')) {
tokens.push_back(token);
}
for (auto t : tokens) {
cout << t << endl;
}
return 0;
}
```
在这里,我们首先将字符串"apple,banana,orange,grape"存储在一个名为str的字符串变量中。然后,我们创建一个名为tokens的字符串向量来存储逗号分割后的单词。接下来,我们使用stringstream将字符串转换为输入流,并使用getline函数从输入流中读取单词,并使用逗号作为分隔符。最后,我们使用for循环遍历tokens向量并打印每个单词。
C++以逗号分割字符串
可以使用stringstream和getline来实现C++中以逗号分割字符串的操作,示例代码如下:
```c++
#include <iostream>
#include <sstream>
#include <string>
int main() {
std::string str = "apple,banana,orange";
std::stringstream ss(str);
std::string token;
while (getline(ss, token, ',')) {
std::cout << token << std::endl;
}
return 0;
}
```
输出结果为:
```
apple
banana
orange
```
在这个例子中,我们首先定义了一个字符串`str`,里面包含了三个水果,每个水果之间用逗号分隔。然后我们使用stringstream将字符串转换成了一个流,接着使用getline函数从流中读取每个水果,并以逗号作为分隔符,将每个水果存储到字符串变量`token`中,并输出`token`的值。最后,我们得到了三个水果的名称。
相关推荐
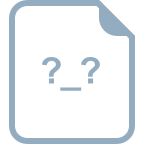












