C语言尝试定义一个这样的结构体: 小明的班级里有若干学生,每个学生都拥有姓名、学号、数学成绩、语文成绩、英语成绩、总分、排名。
时间: 2023-11-04 14:52:48 浏览: 32
你可以这样定义一个类似于这样的结构体:
```c
struct student {
char name[20]; // 姓名
int number; // 学号
float math_score; // 数学成绩
float chinese_score;// 语文成绩
float english_score;// 英语成绩
float total_score; // 总分
int rank; // 排名
};
struct class {
struct student stu[100]; // 班级中的学生,假设不超过100人
int num; // 班级中学生的人数
};
```
这里定义了两个结构体,一个是 `student` 表示学生信息,包括姓名、学号、数学成绩、语文成绩、英语成绩、总分、排名;另一个是 `class` 表示班级信息,包括班级中的学生和学生人数。
相关问题
用C语言编写,定义一个学生结构体类型:姓名身高体重年龄性别学号身份证号
好的,这是一个关于编程的问题,我可以回答。
你可以使用以下代码定义一个学生结构体类型:
```c
typedef struct {
char name[50]; // 姓名
float height; // 身高
float weight; // 体重
int age; // 年龄
char gender; // 性别,可以用 'M' 表示男性,'F' 表示女性
char id[20]; // 学号
char sid[20]; // 身份证号
} Student;
```
这个结构体包含了学生的姓名、身高、体重、年龄、性别、学号和身份证号等信息。你可以根据需求自行修改、添加或删除这些字段。
用C语言,定义一个学生类型的结构体,包括学号 姓名 成绩 定义一个学生变量 在键盘上输入信息 输出学生信息
以下是用C语言定义一个学生类型的结构体,并且在键盘上输入学生信息并输出的示例代码:
```c
#include <stdio.h>
struct student {
int id;
char name[20];
float score;
};
int main() {
struct student s;
printf("请输入学生信息:\n");
printf("学号:");
scanf("%d", &s.id);
printf("姓名:");
scanf("%s", s.name);
printf("成绩:");
scanf("%f", &s.score);
printf("\n学生信息如下:\n");
printf("学号:%d\n", s.id);
printf("姓名:%s\n", s.name);
printf("成绩:%.2f\n", s.score);
return 0;
}
```
运行结果:
```
请输入学生信息:
学号:1001
姓名:张三
成绩:89.5
学生信息如下:
学号:1001
姓名:张三
成绩:89.50
```
相关推荐
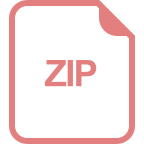
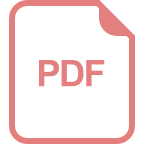
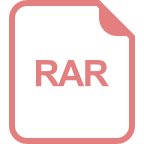
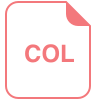
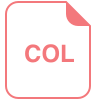
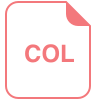
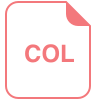
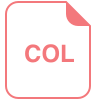







