<template> <div> <div> <span>{{ countdown.days }}</span >天 <span>{{ countdown.hours }}</span >时 <span>{{ countdown.minutes }}</span >分 <span>{{ countdown.seconds }}</span >秒 </div> </div> </template> <script> export default { name: 'WebIndex', data() { return { endTime: '2023-07-04 00:00:00', // 倒计时结束时间 countdown: {}, // 定义响应式数据 }; }, methods: { updateTime() { let now = new Date().getTime(); // 获取当前时间戳 let end = new Date(this.endTime).getTime(); // 获取倒计时结束时间戳 let diff = end - now; // 计算时间差 // 计算天、时、分、秒 let days = Math.floor(diff / (1000 * 60 * 60 * 24)); let hours = Math.floor((diff % (1000 * 60 * 60 * 24)) / (1000 * 60 * 60)); let minutes = Math.floor((diff % (1000 * 60 * 60)) / (1000 * 60)); let seconds = Math.floor((diff % (1000 * 60)) / 1000); // 更新响应式数据 this.countdown = { days: days, hours: hours, minutes: minutes, seconds: seconds, }; }, }, created() { this.updateTime(); // 初始化倒计时 setInterval(() => { this.updateTime(); // 更新倒计时 }, 1000); }, }; </script> <style lang="less" scoped> </style>实现时间差为0的时候清除倒计时
时间: 2024-03-11 17:51:05 浏览: 147
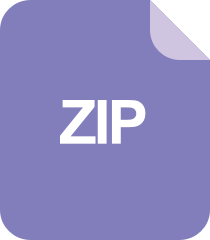
jquery.countdown.js自定义倒计时代码
可以在 `updateTime()` 方法中添加判断,当时间差为 0 时清除定时器,即可实现时间差为 0 的时候清除倒计时。具体实现代码如下:
```
updateTime() {
let now = new Date().getTime(); // 获取当前时间戳
let end = new Date(this.endTime).getTime(); // 获取倒计时结束时间戳
let diff = end - now; // 计算时间差
if (diff <= 0) { // 当时间差为 0 时清除定时器
clearInterval(this.timer);
return;
}
// 计算天、时、分、秒
let days = Math.floor(diff / (1000 * 60 * 60 * 24));
let hours = Math.floor((diff % (1000 * 60 * 60 * 24)) / (1000 * 60 * 60));
let minutes = Math.floor((diff % (1000 * 60 * 60)) / (1000 * 60));
let seconds = Math.floor((diff % (1000 * 60)) / 1000);
// 更新响应式数据
this.countdown = {
days: days,
hours: hours,
minutes: minutes,
seconds: seconds,
};
},
created() {
this.updateTime(); // 初始化倒计时
this.timer = setInterval(() => {
this.updateTime(); // 更新倒计时
}, 1000);
},
```
阅读全文
相关推荐
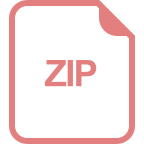
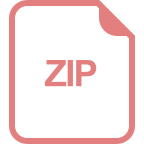


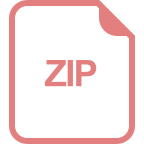
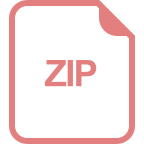
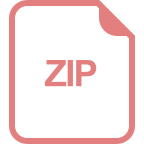
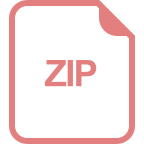
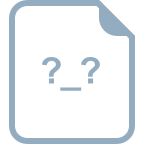
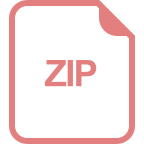