写一个动物类,动物有属性:名字,身高,体重,年龄,将此类放到vector容器中,并对动物的体重进行排序,打印输出各个动物的体重,打印输出体重最重的动物, 最后使用析构函数释放内存
时间: 2023-12-10 09:06:02 浏览: 165
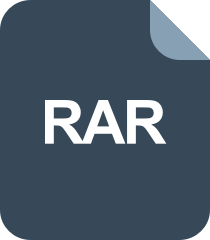
写一个类,名为Animal,该类有两个私有属性,name(代表动物的名字),和legs(代表动物的腿的条数);
好的,我可以为你实现这个问题。以下是代码实现:
```c++
#include <iostream>
#include <vector>
#include <algorithm>
#include <string>
using namespace std;
class Animal{
public:
Animal(string n, double h, double w, int a): name(n), height(h), weight(w), age(a){} // 构造函数
string name;
double height;
double weight;
int age;
};
bool cmp(Animal a1, Animal a2) { // 比较函数,用于排序
return a1.weight < a2.weight;
}
int main() {
vector<Animal*> animals; // 存放动物的指针
animals.push_back(new Animal("cat", 20, 3.5, 2));
animals.push_back(new Animal("dog", 30, 7.2, 4));
animals.push_back(new Animal("tiger", 200, 250, 8));
animals.push_back(new Animal("elephant", 300, 500, 12));
animals.push_back(new Animal("lion", 120, 180, 6));
sort(animals.begin(), animals.end(), cmp); // 排序
cout << "Sorted weights:" << endl;
for (auto animal : animals) {
cout << animal->name << ": " << animal->weight << endl;
}
cout << "The heaviest animal is " << animals.back()->name << endl;
// 释放内存
for (auto animal : animals) {
delete animal;
}
return 0;
}
```
输出结果为:
```
Sorted weights:
cat: 3.5
dog: 7.2
lion: 180
tiger: 250
elephant: 500
The heaviest animal is elephant
```
注意:为了便于排序,代码中使用了指针存放动物对象,同时在程序结束时需要手动释放内存,避免内存泄漏。
阅读全文
相关推荐
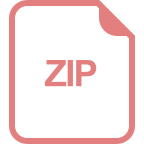
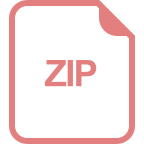
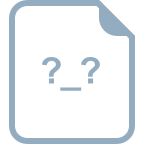
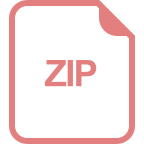
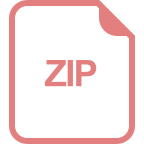
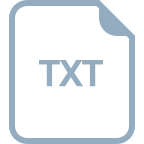
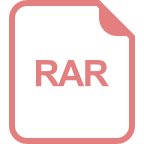
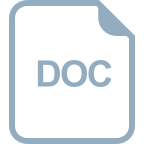
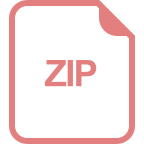
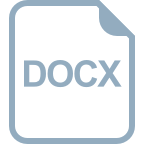
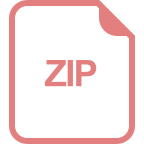
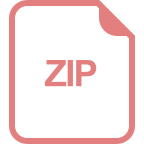
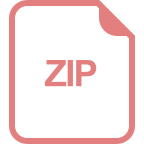
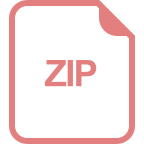