java创建一个aws桶
时间: 2023-08-13 16:17:21 浏览: 49
要在Java中创建AWS S3存储桶(Bucket),需要使用Amazon S3 SDK for Java。以下是一个简单的示例代码:
```java
import com.amazonaws.AmazonClientException;
import com.amazonaws.AmazonServiceException;
import com.amazonaws.auth.profile.ProfileCredentialsProvider;
import com.amazonaws.regions.Region;
import com.amazonaws.regions.Regions;
import com.amazonaws.services.s3.AmazonS3;
import com.amazonaws.services.s3.AmazonS3Client;
import com.amazonaws.services.s3.model.Bucket;
public class CreateBucket {
public static void main(String[] args) throws Exception {
/*
* The ProfileCredentialsProvider will return your [default] credential profile by
* reading from the credentials file located at (~/.aws/credentials).
*/
ProfileCredentialsProvider credentialsProvider = new ProfileCredentialsProvider();
try {
credentialsProvider.getCredentials();
} catch (Exception e) {
throw new AmazonClientException(
"Cannot load the credentials from the credential profiles file. " +
"Please make sure that your credentials file is at the correct " +
"location (~/.aws/credentials), and is in valid format.",
e);
}
AmazonS3 s3 = new AmazonS3Client(credentialsProvider);
Region usWest2 = Region.getRegion(Regions.US_WEST_2);
s3.setRegion(usWest2);
String bucketName = "my-first-s3-bucket-" + System.currentTimeMillis();
try {
System.out.println("Creating bucket " + bucketName + "\n");
s3.createBucket(bucketName);
} catch (AmazonServiceException ase) {
System.out.println("Caught an AmazonServiceException, which means your request made it " +
"to AWS S3, but was rejected with an error response for some reason.");
System.out.println("Error Message: " + ase.getMessage());
System.out.println("HTTP Status Code: " + ase.getStatusCode());
System.out.println("AWS Error Code: " + ase.getErrorCode());
System.out.println("Error Type: " + ase.getErrorType());
System.out.println("Request ID: " + ase.getRequestId());
} catch (AmazonClientException ace) {
System.out.println("Caught an AmazonClientException, which means the client encountered " +
"a serious internal problem while trying to communicate with AWS S3, " +
"such as not being able to access the network.");
System.out.println("Error Message: " + ace.getMessage());
}
}
}
```
在这个例子中,我们使用了AWS SDK for Java提供的AmazonS3客户端来创建一个S3 Bucket。首先,我们需要使用AWS凭证来实例化AmazonS3客户端,然后设置AWS区域(Region)。接着,我们指定要创建的Bucket名称,并使用AmazonS3客户端的createBucket方法来创建Bucket。如果创建Bucket遇到错误,我们会捕获AmazonServiceException和AmazonClientException异常并打印错误信息。
注意:在使用AWS SDK for Java之前,需要先在机器上安装AWS CLI并配置AWS凭证。例如,可以使用aws configure命令来配置AWS凭证。
相关推荐
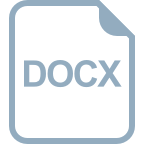
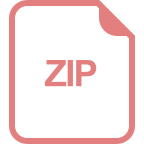
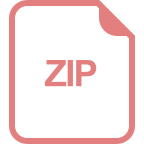














