python 连接海康摄像机,并获取视频中的车辆,统计车辆数量
时间: 2024-04-10 13:27:09 浏览: 11
要连接海康摄像机并获取视频中的车辆,并统计车辆数量,你可以使用海康SDK和OpenCV库来实现。以下是一个简单的示例代码:
```python
from hikvisionapi import Client # 海康SDK库
import cv2
# 海康摄像机登录
def login(username, password, ip):
client = Client(ip, username, password)
client.login()
return client
# 获取视频流
def get_video_stream(client):
stream = client.get_stream()
return stream
# 统计车辆数量
def count_vehicles(stream):
vehicle_cascade = cv2.CascadeClassifier('vehicle.xml') # 车辆检测模型,可根据实际情况替换
vehicle_count = 0
while True:
frame = stream.get_frame() # 获取视频帧
gray = cv2.cvtColor(frame, cv2.COLOR_BGR2GRAY)
vehicles = vehicle_cascade.detectMultiScale(gray, scaleFactor=1.1, minNeighbors=5, minSize=(30, 30))
for (x, y, w, h) in vehicles:
cv2.rectangle(frame, (x, y), (x+w, y+h), (0, 255, 0), 2)
vehicle_count += 1
cv2.imshow('Video', frame)
if cv2.waitKey(1) & 0xFF == ord('q'):
break
cv2.destroyAllWindows()
return vehicle_count
# 主函数
def main():
username = 'your_username' # 替换为实际的摄像机用户名
password = 'your_password' # 替换为实际的摄像机密码
ip = 'camera_ip_address' # 替换为实际的摄像机IP地址
client = login(username, password, ip)
stream = get_video_stream(client)
vehicle_count = count_vehicles(stream)
print('车辆数量:', vehicle_count)
if __name__ == '__main__':
main()
```
上述示例中,`login`函数用于登录海康摄像机,你需要替换`username`、`password`和`ip`为实际的摄像机登录信息。`get_video_stream`函数用于获取视频流,`count_vehicles`函数使用OpenCV的级联分类器来检测车辆并统计数量。请确保你已经安装了`hikvisionapi`和`opencv-python`库。
注意:在使用前,请先下载并将Haar级联分类器XML文件(如车辆检测模型)放在同一目录下,或者根据实际情况修改路径。
希望对你有帮助!如有任何问题,请随时提问。
相关推荐
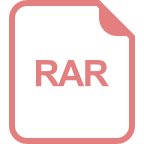
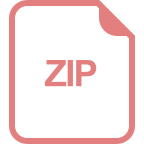














