Traceback (most recent call last): File "i:\18Breakageratecalculation\SVM run\mergeslic", line 20, in <module> x, y = map(int, filename.split("_")[2:4]) ^^^^ ValueError: not enough values to unpack (expected 2, got 0)
时间: 2024-03-23 07:39:46 浏览: 11
这个错误通常表示在使用 `split()` 函数时,分割的结果不符合预期,导致解包(unpack)时出现了错误。
根据你提供的错误信息,这个错误发生在第20行,这个行的代码是:
```python
x, y = map(int, filename.split("_")[2:4])
```
根据错误信息,这行代码的问题可能在于 `filename.split("_")[2:4]` 返回的结果不足两个元素,无法解包成 `x` 和 `y` 两个变量。
为了确定问题的原因,可以在这行代码前面添加一行代码,打印出 `filename.split("_")` 的结果,看看它是否符合预期。例如:
```python
print(filename.split("_"))
x, y = map(int, filename.split("_")[2:4])
```
如果输出结果不符合预期,可以检查文件名的格式是否正确,以及 `split()` 函数的参数是否正确。如果输出结果符合预期,可以检查 `map()` 函数的结果是否正确,以及 `x` 和 `y` 是否已经被定义过。
相关问题
Traceback (most recent call last): File "i:\18Breakageratecalculation\SVM run\hog-color-svm", line 11, in <module> for i in range(num_samples = 681): ^^^^^^^^^^^^^^^^^^^^^^^^ TypeError: range() takes no keyword arguments
在`range()`函数中不能使用关键字参数来指定循环次数,因此抛出了`TypeError: range() takes no keyword arguments`异常。你需要将`num_samples = 681`这个赋值语句移到循环外面,然后使用`range(num_samples)`来指定循环次数。例如,你可以这样修改代码:
```
import cv2
import numpy as np
# 提取图像的HOG特征
def get_hog_features(image):
hog = cv2.HOGDescriptor()
hog_features = hog.compute(image)
return hog_features
# 加载训练数据集
train_data = [r"I:\18Breakageratecalculation\SVM run\detection_cut\whole\train128"]
train_labels = [r"I:\18Breakageratecalculation\SVM run\detection_cut\whole\train128\labels.txt"]
num_samples = 681 # 定义循环次数
for i in range(num_samples):
image = cv2.imread('image_'+str(i)+'.jpg', 0)
hog_features = get_hog_features(image)
hsv_image = cv2.cvtColor(image, cv2.COLOR_BGR2HSV)
color_hist = cv2.calcHist([hsv_image], [0, 1], None, [180, 256], [0, 180, 0, 256])
color_features = cv2.normalize(color_hist, color_hist).flatten()
train_data.append(hog_features)
train_labels.append(labels[i])
# 训练SVM模型
svm = cv2.ml.SVM_create()
svm.setType(cv2.ml.SVM_C_SVC)
svm.setKernel(cv2.ml.SVM_LINEAR)
svm.train(np.array(train_data), cv2.ml.ROW_SAMPLE, np.array(train_labels))
# 对测试图像进行分类
test_image = cv2.imread('I:\18Breakageratecalculation\mask-slic use\maskSLIC-master\result\split\result2\maskslic2_roi.png', 0)
test_features = get_hog_features(test_image)
result = svm.predict(test_features.reshape(1,-1))
# 显示分割结果
result_image = np.zeros(test_image.shape, np.uint8)
for i in range(test_image.shape[0]):
for j in range(test_image.shape[1]):
if result[i,j] == 1:
result_image[i,j] = 255
cv2.imshow('I:\18Breakageratecalculation\mask-slic use\maskSLIC-master\result\split\result2\Result.png', result_image)
cv2.waitKey(0)
cv2.destroyAllWindows()
```
Traceback (most recent call last): File "i:\18Breakageratecalculation\SVM run\mergeslic", line 24, in <module> img.paste(new_img, (0, 0), new_img) File "C:\Users\win10\AppData\Local\Programs\Python\Python311\Lib\site-packages\PIL\Image.py", line 1732, in paste self.im.paste(im, box, mask.im) ValueError: bad transparency mask
这个错误通常是由于你尝试将不透明的图像用作透明度掩码。在你的代码中,你将 `new_img` 作为透明度掩码传递给了 `img.paste()` 方法。但是,`new_img` 是一个不透明的图像,而不是一个透明度掩码。因此,你需要创建一个透明度掩码,或者使用一个透明的图像作为透明度掩码。
以下是一个示例代码,可以将0类转换为蓝色,2类转换为黑色,1类转换为红色,并使用一个透明度掩码将颜色应用到原始图像:
```
from PIL import Image
img_path = "001.png"
class_label = 0
# Open the image
img = Image.open(img_path)
# Define the color mapping
color_map = {
0: (0, 0, 255), # Blue
1: (255, 0, 0), # Red
2: (0, 0, 0) # Black
}
# Convert the class label to a color
color = color_map.get(class_label, (0, 0, 0))
# Create a new image with the color
new_img = Image.new('RGBA', img.size, color+(0,))
# Paste the new image onto the original image
img.paste(new_img, (0, 0), new_img)
# Save the image
img.save("colored.png")
```
这个代码将创建一个透明度掩码,然后将颜色应用到原始图像。注意,我们使用了 `Image.new('RGBA', img.size, color+(0,))` 来创建一个透明的图像,而不是不透明的图像。最后的 `(0,)` 是用来添加透明度通道的。
相关推荐
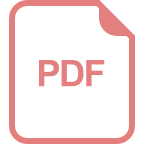
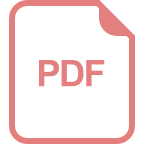
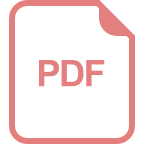






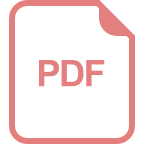
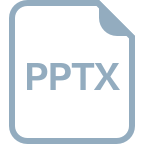
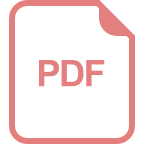
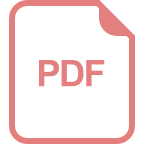
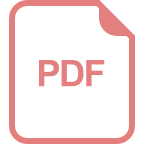
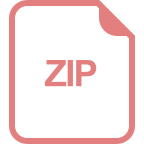
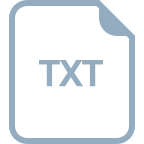
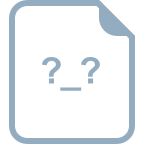