基于机器学习的水果识别的水果集处理python代码实现
时间: 2023-12-25 22:05:17 浏览: 32
以下是基于机器学习的水果识别的水果集处理Python代码实现:
```python
import numpy as np
import cv2
import os
from sklearn.model_selection import train_test_split
from keras.utils import np_utils
from keras.models import Sequential
from keras.layers import Conv2D, MaxPooling2D, Flatten, Dense
# 构建数据集
def load_dataset(path):
fruits = []
labels = []
for fruit_dir in os.listdir(path):
fruit_label = fruit_dir.split("_")[0]
fruit_path = os.path.join(path, fruit_dir)
for fruit_image in os.listdir(fruit_path):
img_path = os.path.join(fruit_path, fruit_image)
img = cv2.imread(img_path)
img = cv2.resize(img, (100, 100))
fruits.append(img)
labels.append(fruit_label)
return fruits, labels
# 加载数据集
fruits, labels = load_dataset("fruits")
X_train, X_test, y_train, y_test = train_test_split(fruits, labels, test_size=0.2, random_state=42)
# 数据预处理
num_classes = len(set(labels))
X_train = np.array(X_train).astype('float32') / 255
X_test = np.array(X_test).astype('float32') / 255
y_train = np_utils.to_categorical(np.array(y_train), num_classes)
y_test = np_utils.to_categorical(np.array(y_test), num_classes)
# 搭建卷积神经网络模型
model = Sequential()
model.add(Conv2D(32, (3, 3), activation='relu', input_shape=(100, 100, 3)))
model.add(MaxPooling2D(pool_size=(2, 2)))
model.add(Conv2D(64, (3, 3), activation='relu'))
model.add(MaxPooling2D(pool_size=(2, 2)))
model.add(Flatten())
model.add(Dense(128, activation='relu'))
model.add(Dense(num_classes, activation='softmax'))
# 编译模型
model.compile(loss='categorical_crossentropy', optimizer='adam', metrics=['accuracy'])
# 训练模型
model.fit(X_train, y_train, validation_data=(X_test, y_test), epochs=10, batch_size=32)
# 评估模型
score = model.evaluate(X_test, y_test)
print('Test loss:', score[0])
print('Test accuracy:', score[1])
```
以上代码实现了对水果集进行预处理,搭建卷积神经网络模型,以及训练和评估模型的过程。通过这个代码,可以实现对水果图片进行分类识别。
相关推荐
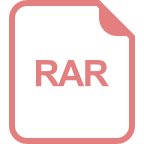
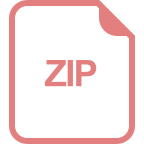














