matlab中的Traing、Validation、Testing
时间: 2023-07-04 09:10:48 浏览: 106
在MATLAB中,Traing、Validation、Testing是机器学习中常用的三种数据集划分方式,用于训练、验证和测试模型的性能。具体解释如下:
1. Training数据集:用于训练模型的数据集,通常占总数据集的70%~80%左右。通过对训练数据集的学习,模型可以调整自己的参数,提高对数据的拟合能力。
2. Validation数据集:用于验证模型的泛化能力和调参的数据集,通常占总数据集的10%~20%左右。在训练过程中,通过对验证数据集的评估,可以确定模型是否过拟合或欠拟合,并进行相应的调整。
3. Testing数据集:用于测试模型的性能和泛化能力的数据集,通常占总数据集的10%~20%左右。在训练和验证完成后,通过对测试数据集的评估,可以对模型的性能进行最终的评价。
在使用这三种数据集时,需要注意以下几点:
1. 数据集划分要随机,避免因数据集划分不合理而导致模型性能不稳定。
2. 数据集之间应该互不重叠,避免模型在训练、验证和测试时重复使用相同的数据。
3. 数据集的大小和比例应该根据具体问题进行合理选择,尽可能保证数据集的多样性和代表性。
相关问题
tokenizer = Tokenizer(num_words=max_words) tokenizer.fit_on_texts(data['text']) sequences = tokenizer.texts_to_sequences(data['text']) word_index = tokenizer.word_index print('Found %s unique tokens.' % len(word_index)) data = pad_sequences(sequences,maxlen=maxlen) labels = np.array(data[:,:1]) print('Shape of data tensor:',data.shape) print('Shape of label tensor',labels.shape) indices = np.arange(data.shape[0]) np.random.shuffle(indices) data = data[indices] labels = labels[indices] x_train = data[:traing_samples] y_train = data[:traing_samples] x_val = data[traing_samples:traing_samples+validation_samples] y_val = data[traing_samples:traing_samples+validation_samples] model = Sequential() model.add(Embedding(max_words,100,input_length=maxlen)) model.add(Flatten()) model.add(Dense(32,activation='relu')) model.add(Dense(10000,activation='sigmoid')) model.summary() model.compile(optimizer='rmsprop', loss='binary_crossentropy', metrics=['acc']) history = model.fit(x_train,y_train, epochs=1, batch_size=128, validation_data=[x_val,y_val]) import matplotlib.pyplot as plt acc = history.history['acc'] val_acc = history.history['val_acc'] loss = history.history['loss'] val_loss = history.history['val_loss'] epoachs = range(1,len(acc) + 1) plt.plot(epoachs,acc,'bo',label='Training acc') plt.plot(epoachs,val_acc,'b',label = 'Validation acc') plt.title('Training and validation accuracy') plt.legend() plt.figure() plt.plot(epoachs,loss,'bo',label='Training loss') plt.plot(epoachs,val_loss,'b',label = 'Validation loss') plt.title('Training and validation loss') plt.legend() plt.show() max_len = 10000 x_train = keras.preprocessing.sequence.pad_sequences(x_train, maxlen=max_len) x_test = data[10000:,0:] x_test = keras.preprocessing.sequence.pad_sequences(x_test, maxlen=max_len) # 将标签转换为独热编码 y_train = np.eye(2)[y_train] y_test = data[10000:,:1] y_test = np.eye(2)[y_test]
这段代码中存在几个问题:
1. `labels` 的赋值有误。应该是 `labels = np.array(data[:, 1:])`,因为标签是从第二列开始的。
2. `validation_data` 的格式有误。应该是 `validation_data=(x_val, y_val)`,而不是 `validation_data=[x_val, y_val]`。
3. 在使用 `Sequential` 模型时,需要指定输入的维度。这里应该是 `model.add(Dense(10000, activation='sigmoid', input_dim=maxlen))`,而不是 `model.add(Dense(10000, activation='sigmoid'))`。
4. 绘制图表时,应该使用 `val_acc` 和 `val_loss`,而不是 `test_acc` 和 `test_loss`。
5. 在将标签转换为独热编码时,应该使用 `y_train[:, 0]` 和 `y_test[:, 0]`,而不是 `y_train` 和 `y_test`。
以下是修改后的代码:
```python
tokenizer = Tokenizer(num_words=max_words)
tokenizer.fit_on_texts(data['text'])
sequences = tokenizer.texts_to_sequences(data['text'])
word_index = tokenizer.word_index
print('Found %s unique tokens.' % len(word_index))
data = pad_sequences(sequences, maxlen=maxlen)
labels = np.array(data[:, 1:])
print('Shape of data tensor:', data.shape)
print('Shape of label tensor', labels.shape)
indices = np.arange(data.shape[0])
np.random.shuffle(indices)
data = data[indices]
labels = labels[indices]
x_train = data[:training_samples]
y_train = labels[:training_samples]
x_val = data[training_samples:training_samples+validation_samples]
y_val = labels[training_samples:training_samples+validation_samples]
model = Sequential()
model.add(Embedding(max_words, 100, input_length=maxlen))
model.add(Flatten())
model.add(Dense(32, activation='relu'))
model.add(Dense(10000, activation='sigmoid', input_dim=maxlen))
model.summary()
model.compile(optimizer='rmsprop',
loss='binary_crossentropy',
metrics=['acc'])
history = model.fit(x_train, y_train,
epochs=1,
batch_size=128,
validation_data=(x_val, y_val))
import matplotlib.pyplot as plt
acc = history.history['acc']
val_acc = history.history['val_acc']
loss = history.history['loss']
val_loss = history.history['val_loss']
epochs = range(1, len(acc) + 1)
plt.plot(epochs, acc, 'bo', label='Training acc')
plt.plot(epochs, val_acc, 'b', label='Validation acc')
plt.title('Training and validation accuracy')
plt.legend()
plt.figure()
plt.plot(epochs, loss, 'bo', label='Training loss')
plt.plot(epochs, val_loss, 'b', label='Validation loss')
plt.title('Training and validation loss')
plt.legend()
plt.show()
max_len = 10000
x_train = keras.preprocessing.sequence.pad_sequences(x_train, maxlen=max_len)
x_test = data[10000:, 0:]
x_test = keras.preprocessing.sequence.pad_sequences(x_test, maxlen=max_len)
# 将标签转换为独热编码
y_train = np.eye(2)[y_train[:, 0]]
y_test = data[10000:, 1:]
y_test = np.eye(2)[y_test[:, 0]]
```
ValueError: [x] Muiti-speaker traing error : spk_id must be a positive integer from 1 to n_spk
我看到你的问题是一个 Python 错误,它似乎是在进行多说话者训练时出现的。错误提示说 spk_id 必须是从 1 到 n_spk 的正整数。我建议你检查你的代码,确认 spk_id 是否在这个范围内,并且确保它是一个整数。你还可以查看你的训练数据,检查说话者标识符是否正确,并且没有缺失或冗余的数据。如果你需要更多的帮助,请提供更多的上下文和代码,这样我才能更好地帮助你解决这个问题。
阅读全文
相关推荐
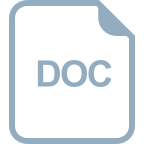
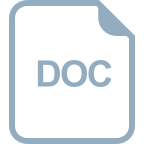
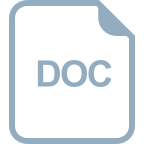
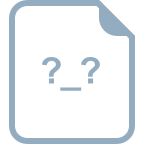
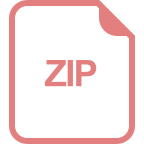
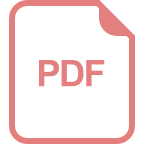
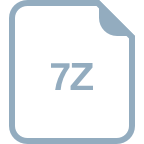
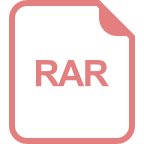
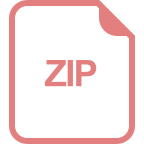


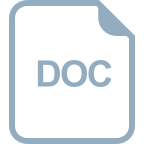
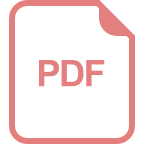
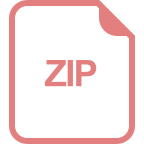