python 使用cookies 发送post请求 获取结果
时间: 2023-06-26 10:03:49 浏览: 65
可以使用 Python 的 requests 库来发送带有 Cookies 的 POST 请求,并获取响应结果。
首先,你需要获取 Cookies,可以使用 requests 库发送 GET 请求,然后通过 response.cookies 获取:
```python
import requests
url = "https://example.com/login"
response = requests.get(url)
cookies = response.cookies
```
接着,你可以使用 Cookies 发送 POST 请求,例如:
```python
import requests
url = "https://example.com/data"
data = {"key": "value"}
cookies = {"session_id": "12345"}
response = requests.post(url, data=data, cookies=cookies)
print(response.text)
```
在这个例子中,我们使用了 requests 库的 post 方法发送一个 POST 请求,其中包含了一些数据 data 和 Cookies。最后,我们打印了响应结果 response.text。
需要注意的是,Cookies 应该是一个字典,键值对表示了 Cookie 的名称和值。在这个例子中,我们使用了一个名为 "session_id" 的 Cookie。你需要根据实际情况替换为你自己的 Cookie 名称和值。
相关问题
python带cookies发送post请求_Python模仿POST提交HTTP数据及使用Cookie值的方法
在Python中发送带有cookies的POST请求,可以使用requests库。具体步骤如下:
1. 安装requests库
在命令行中输入以下命令安装requests库:
```
pip install requests
```
2. 导入requests库
在Python代码中导入requests库:
```python
import requests
```
3. 构造请求参数
构造POST请求参数,可以使用字典类型。例如:
```python
params = {
'username': 'your_username',
'password': 'your_password'
}
```
4. 设置cookies
设置cookies,也可以使用字典类型。例如:
```python
cookies = {
'cookie_name': 'cookie_value'
}
```
5. 发送POST请求
使用requests库发送POST请求,并传入参数和cookies。例如:
```python
response = requests.post(url, data=params, cookies=cookies)
```
其中,url是请求的URL地址。
6. 获取响应数据
获取响应数据,可以使用response对象的text属性。例如:
```python
print(response.text)
```
完整代码示例:
```python
import requests
url = 'http://example.com/login'
params = {
'username': 'your_username',
'password': 'your_password'
}
cookies = {
'cookie_name': 'cookie_value'
}
response = requests.post(url, data=params, cookies=cookies)
print(response.text)
```
注意:在实际使用中,需要替换掉示例代码中的URL、参数和cookies值。
python带cookies的post请求
### 回答1:
Python带cookies的post请求可以通过以下步骤实现:
1. 导入requests库
2. 创建一个session对象,该对象可以自动保存cookies
3. 构造post请求的参数和headers
4. 发送post请求,并将返回的结果保存在一个变量中
5. 打印结果
示例代码如下:
```
import requests
# 创建一个session对象
session = requests.session()
# 构造post请求的参数和headers
url = 'http://example.com/login'
data = {'username': 'user', 'password': 'pass'}
headers = {'User-Agent': 'Mozilla/5. (Windows NT 10.; Win64; x64) AppleWebKit/537.36 (KHTML, like Gecko) Chrome/58..3029.110 Safari/537.3'}
# 发送post请求,并将返回的结果保存在一个变量中
response = session.post(url, data=data, headers=headers)
# 打印结果
print(response.text)
```
在上面的代码中,我们使用了session对象来发送post请求,并将返回的结果保存在response变量中。由于session对象自动保存了cookies,因此我们可以在后续的请求中继续使用该session对象,从而实现带cookies的请求。
### 回答2:
在Python中,如果需要进行带cookie的POST请求,可以使用requests模块。该模块提供了很多HTTP请求相关的方法,可以轻松地进行访问和交互。
步骤如下:
1. 导入requests模块
首先需要导入requests模块。在Python中,使用import语句来导入一个模块。将requests导入进来:
import requests
2. 准备请求头和请求体
在进行POST请求时,需要设置请求头和请求体。如果需要设置cookies,可以将cookies添加到请求头中。可以使用requests中的cookies属性来设置,示例代码如下:
headers = {
'Content-Type': 'application/json', # 请求头设置为json格式
'Cookie': 'session_id=1234567890' # 设置cookies
}
data = {
'name': '张三',
'age': 20
}
3. 发送POST请求
准备好请求头和请求体之后,就可以发送POST请求了。可以使用requests模块中的post方法来发送请求。该方法的参数包括url、请求头、请求体等信息。
url = 'http://www.example.com/api/user'
response = requests.post(url, headers=headers, data=json.dumps(data))
print(response.text)
在上面的示例代码中,url是请求的地址,headers包含请求头,data是请求体。post方法返回的是一个response对象,可以使用response.text属性获取返回的响应内容。
总结:
以上就是Python中使用requests模块进行带cookies的POST请求的步骤。通过设置请求头和请求体,可以轻松地发送POST请求并接收服务器的响应。requests是一个十分方便实用的HTTP库,推荐使用。
### 回答3:
Python带有Cookies的POST请求是使用Python编写的代码发送包含Cookies的POST请求。Cookies是网站在用户浏览器中存储的一些数据,用于跟踪用户会话和其他用途。
在Python中,我们可以使用requests库来发送HTTP请求。通过设置requests.post()函数的cookies参数,可以在POST请求中传递cookies。以下是一个简单的Python代码示例:
import requests
cookies = {"user_id": "123456"}
payload = {"name": "John Doe", "age": 30}
response = requests.post("http://www.example.com/post_data", data=payload, cookies=cookies)
print(response.text)
在上面的代码中,我们首先定义了一个名为cookies的字典,包含了要传递的cookies值。然后定义了名为payload的字典,包含了POST请求要发送的参数数据。使用requests.post()函数发送POST请求,其中传递了数据和cookies参数。最后,通过response.text属性获取响应内容。
需要注意的是,cookies参数必须是一个字典,键和值都是字符串。如果需要传递多个cookies,需要在字典中添加多个键值对。另外,当cookies被设置时,可以通过response.cookies属性获取服务器返回的所有cookies值,可以使用requests.utils.dict_from_cookiejar()函数把cookies内容导出为字典形式。
总的来说,Python带有Cookies的POST请求是非常常见的网络请求方式。使用Python编写代码,可以轻松的通过requests库发送带有Cookies的POST请求,方便实用,适用于各种场景。
相关推荐
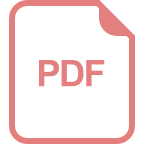
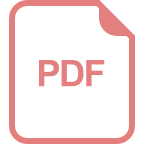
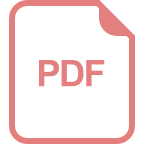












