优化这段代码
时间: 2023-08-11 09:24:25 浏览: 39
这段代码可以进行一些优化,例如:
1. 使用动态分配的缓冲区代替固定长度的字符数组,以避免缓冲区溢出的风险。
2. 使用智能指针等RAII技术管理动态分配的内存,以避免内存泄漏和悬空指针的问题。
3. 在读取像素值时,可以使用istream::read函数一次性读取整个图像的像素值,而不是逐个读取每个像素值。
下面是优化后的代码示例:
```c++
#include <iostream>
#include <fstream>
#include <string>
#include <memory>
using namespace std;
int main() {
string filename = "example.ppm";
unique_ptr<char[]> buffer(new char[256]);
ifstream infile(filename, ios::binary);
if (!infile) {
cout << "Failed to open file: " << filename << endl;
return 1;
}
infile.getline(buffer.get(), 256); // 文件类型
infile.getline(buffer.get(), 256); // 注释
infile.getline(buffer.get(), 256); // 图像尺寸
int width, height;
sscanf(buffer.get(), "%d %d", &width, &height);
infile.getline(buffer.get(), 256); // 最大像素值
unique_ptr<unsigned char[]> image(new unsigned char[width * height]);
infile.read(reinterpret_cast<char*>(image.get()), width * height);
infile.close();
// 打印读取到的像素值
for (int i = 0; i < height; i++) {
for (int j = 0; j < width; j++) {
cout << static_cast<int>(image[i * width + j]) << " ";
}
cout << endl;
}
return 0;
}
```
这段代码使用了unique_ptr和动态分配的缓冲区来管理内存,使用了istream::read函数一次性读取像素值,以及使用了static_cast将unsigned char类型的像素值转换为int类型,以便打印和处理。
相关推荐
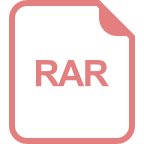
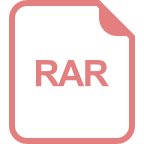
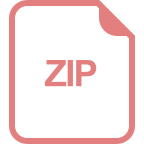


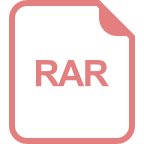
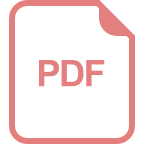
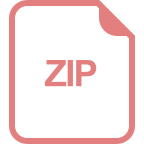
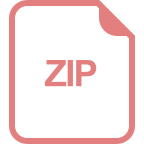
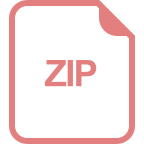
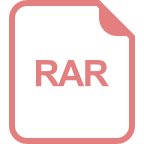
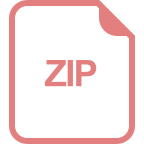
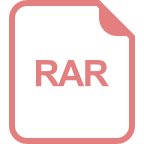
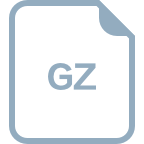
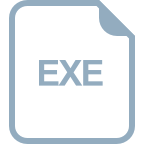
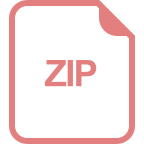