行编写DBSCAN密度聚类算法,绘制数据集的聚类结果
时间: 2023-06-27 08:01:25 浏览: 87
好的,以下是Python代码实现DBSCAN密度聚类算法,并绘制数据集的聚类结果:
```python
import numpy as np
import matplotlib.pyplot as plt
class DBSCAN:
def __init__(self, eps=0.5, min_samples=5):
self.eps = eps
self.min_samples = min_samples
def fit(self, X):
self.X = X
self.n_samples = X.shape[0]
self.visited = np.zeros(self.n_samples, dtype=bool)
self.labels = np.zeros(self.n_samples, dtype=int)
self.n_cluster = 0
for i in range(self.n_samples):
if not self.visited[i]:
self.visited[i] = True
neighbors = self.find_neighbors(i)
if len(neighbors) < self.min_samples:
self.labels[i] = -1
else:
self.expand_cluster(i, neighbors)
self.n_cluster += 1
def find_neighbors(self, i):
return np.where(np.linalg.norm(self.X - self.X[i], axis=1) <= self.eps)[0]
def expand_cluster(self, i, neighbors):
self.labels[i] = self.n_cluster
while len(neighbors) > 0:
j = neighbors[0]
if not self.visited[j]:
self.visited[j] = True
new_neighbors = self.find_neighbors(j)
if len(new_neighbors) >= self.min_samples:
neighbors = np.concatenate((neighbors, new_neighbors))
if self.labels[j] == 0:
self.labels[j] = self.n_cluster
neighbors = neighbors[1:]
def plot(self):
colors = ['red', 'blue', 'green', 'orange', 'purple', 'brown', 'pink', 'gray', 'olive', 'cyan']
for i in range(self.n_samples):
if self.labels[i] == -1:
color = 'black'
else:
color = colors[self.labels[i] % len(colors)]
plt.scatter(self.X[i][0], self.X[i][1], c=color)
plt.show()
```
其中,`eps`表示邻域半径,`min_samples`表示最小样本数。`fit`方法用于拟合数据,`plot`方法用于绘制聚类结果。
接下来,我们使用DBSCAN算法对一个数据集进行聚类,并绘制聚类结果:
```python
from sklearn.datasets import make_moons
# 生成随机数据集
X, y = make_moons(n_samples=200, noise=0.1, random_state=42)
# 使用DBSCAN算法进行聚类
dbscan = DBSCAN(eps=0.3, min_samples=5)
dbscan.fit(X)
# 绘制聚类结果
dbscan.plot()
```
运行以上代码,可以得到以下聚类结果图:

阅读全文
相关推荐
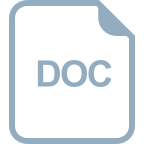
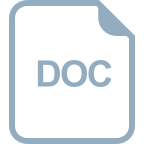
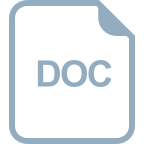


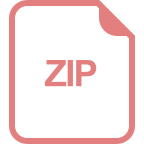
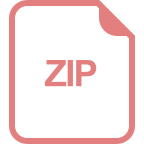




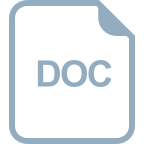
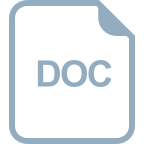
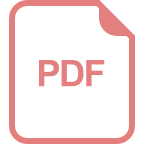
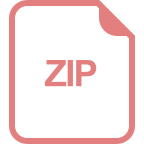
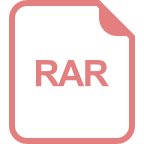
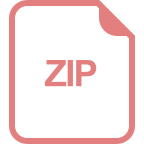
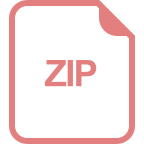
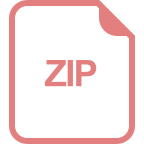