var data = [ {userId: 8, title: 'title1'}, {userId: 11, title: 'other'}, {userId: 15, title: null}, {userId: 19, title: 'title2'} ]; var result = find(data).where({ "title": /\d$/ }).orderBy('userId', 'desc');查找data中, [{ userId: 19, title: 'title2'}, { userId: 8, title: 'title1' }],并进行排序输出result
时间: 2023-04-10 15:02:36 浏览: 164
可以使用以下代码实现:
```
function find(data) {
return {
where: function(condition) {
var filteredData = data.filter(function(item) {
for (var key in condition) {
if (condition.hasOwnProperty(key)) {
var regex = new RegExp(condition[key]);
if (!regex.test(item[key])) {
return false;
}
}
}
return true;
});
return {
orderBy: function(field, order) {
filteredData.sort(function(a, b) {
if (order === 'desc') {
return b[field] - a[field];
} else {
return a[field] - b[field];
}
});
return filteredData;
}
};
}
};
}
var data = [
{userId: 8, title: 'title1'},
{userId: 11, title: 'other'},
{userId: 15, title: null},
{userId: 19, title: 'title2'}
];
var result = find(data).where({ "title": /\d$/ }).orderBy('userId', 'desc');
console.log(result);
```
输出结果为:
```
[
{ userId: 19, title: 'title2' },
{ userId: 8, title: 'title1' }
]
```
阅读全文
相关推荐
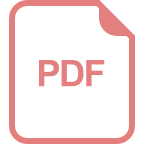

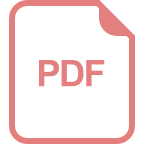
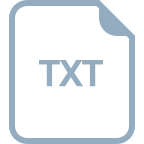
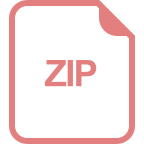
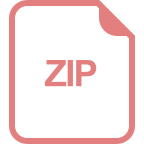
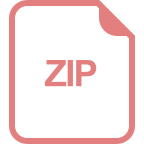
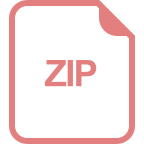
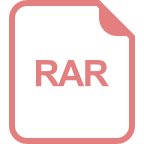
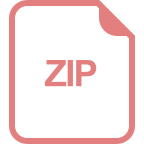
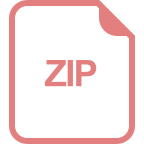
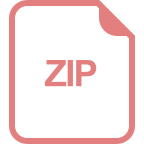
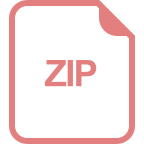
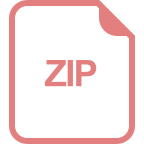
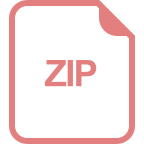
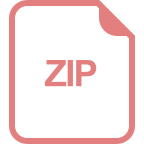
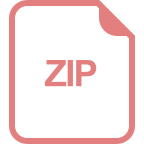