用C语言写出k最近邻分类算法实现代码
时间: 2023-07-05 09:08:11 浏览: 91
以下是用C语言实现k最近邻分类算法的示例代码:
```c
#include <stdio.h>
#include <stdlib.h>
#include <math.h>
typedef struct {
double x;
double y;
int label;
} Point;
int main() {
int k, n;
printf("Enter the value of k: ");
scanf("%d", &k);
printf("Enter the number of points: ");
scanf("%d", &n);
Point* points = (Point*) malloc(n * sizeof(Point));
printf("Enter the coordinates and label of each point:\n");
for (int i = 0; i < n; i++) {
scanf("%lf %lf %d", &points[i].x, &points[i].y, &points[i].label);
}
// Classify new points
int q;
printf("Enter the number of new points to classify: ");
scanf("%d", &q);
printf("Enter the coordinates of each new point:\n");
for (int i = 0; i < q; i++) {
double x, y;
printf("Point %d: ", i+1);
scanf("%lf %lf", &x, &y);
// Compute distance between new point and all points in dataset
double distances[n];
for (int j = 0; j < n; j++) {
double dx = x - points[j].x;
double dy = y - points[j].y;
distances[j] = sqrt(dx*dx + dy*dy);
}
// Find k nearest points
int indices[n];
for (int j = 0; j < n; j++) {
indices[j] = j;
}
for (int j = 0; j < k; j++) {
int min_index = j;
for (int l = j+1; l < n; l++) {
if (distances[l] < distances[min_index]) {
min_index = l;
}
}
double temp_dist = distances[j];
distances[j] = distances[min_index];
distances[min_index] = temp_dist;
int temp_index = indices[j];
indices[j] = indices[min_index];
indices[min_index] = temp_index;
}
// Classify new point by majority vote of k nearest points
int label_counts[3] = {0, 0, 0};
for (int j = 0; j < k; j++) {
int label = points[indices[j]].label;
label_counts[label-1]++;
}
int max_count = 0;
int max_label = 1;
for (int j = 0; j < 3; j++) {
if (label_counts[j] > max_count) {
max_count = label_counts[j];
max_label = j+1;
}
}
printf("Classified as label %d\n", max_label);
}
free(points);
return 0;
}
```
这里实现了一个简单的k最近邻分类器,可以处理二维空间中的点,并将它们分为三个类别。用户可以输入要分类的新点的坐标,并得到它所属的类别。注意,这里假设数据集中有且仅有三个类别,因此在实际应用中可能需要根据具体情况作出调整。
阅读全文
相关推荐
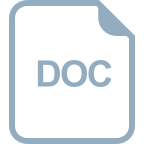
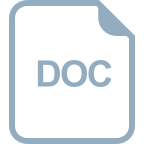
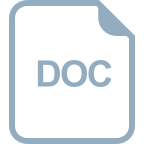
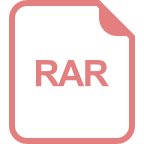
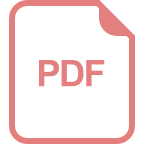
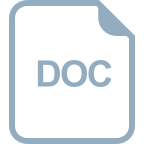
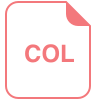
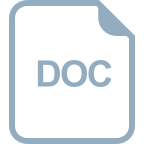
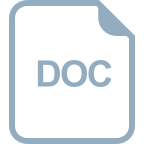
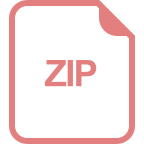
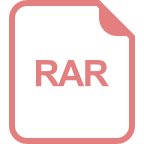