pytorch获取tensor某一维
时间: 2023-10-18 17:12:04 浏览: 97
可以使用 PyTorch 中的索引方式来获取 tensor 的某一维。具体操作为:
```python
import torch
# 创建一个 3x4 的 tensor
tensor = torch.tensor([[1, 2, 3, 4], [5, 6, 7, 8], [9, 10, 11, 12]])
# 获取第2列
col_2 = tensor[:, 1]
print(col_2)
```
输出结果为:
```
tensor([ 2, 6, 10])
```
在上面的例子中,`[:, 1]` 表示获取 tensor 的所有行,第 1 列的元素。如果要获取其他维度的元素,只需要在索引中指定相应的维度即可。例如:
```python
# 获取第2行
row_2 = tensor[1, :]
print(row_2)
```
输出结果为:
```
tensor([5, 6, 7, 8])
```
相关问题
如何获取tensor某一维数据
你可以使用 PyTorch 中的索引操作来获取tensor的某一维数据。以下是一些示例:
1. 获取第一维的数据:
```python
import torch
# 创建一个3维的tensor
x = torch.tensor([[[1, 2], [3, 4]], [[5, 6], [7, 8]], [[9, 10], [11, 12]]])
# 获取第一维的数据
x_0 = x[0]
print(x_0)
```
输出:
```
tensor([[1, 2],
[3, 4]])
```
2. 获取第二维的数据:
```python
import torch
# 创建一个3维的tensor
x = torch.tensor([[[1, 2], [3, 4]], [[5, 6], [7, 8]], [[9, 10], [11, 12]]])
# 获取第二维的数据
x_1 = x[:, 0, :]
print(x_1)
```
输出:
```
tensor([[ 1, 2],
[ 5, 6],
[ 9, 10]])
```
3. 获取第三维的数据:
```python
import torch
# 创建一个3维的tensor
x = torch.tensor([[[1, 2], [3, 4]], [[5, 6], [7, 8]], [[9, 10], [11, 12]]])
# 获取第三维的数据
x_2 = x[:, :, 0]
print(x_2)
```
输出:
```
tensor([[ 1, 3],
[ 5, 7],
[ 9, 11]])
```
在上述示例中,我们使用了不同的索引操作来获取tensor的不同维数据。使用这些操作,你可以轻松地在PyTorch中获取tensor的任何维数据。
pytorch获取tensor平均值
在PyTorch中,如果你想获取一个Tensor的平均值,你可以使用`.mean()`函数。如果你想要全局平均值(在整个Tensor上计算),可以这样做:
```python
import torch
# 假设 tensor 是一个 PyTorch 的 Tensor
example_tensor = torch.tensor([[1, 2, 3], [4, 5, 6]])
average_value = example_tensor.mean()
# 或者,如果你想要沿着特定维度求平均,可以指定 dim 参数,如按行或按列求均值
row_average = example_tensor.mean(dim=0)
column_average = example_tensor.mean(dim=1)
print("全局平均值:", average_value)
print("按行平均值:", row_average)
print("按列平均值:", column_average)
```
上述代码首先导入了`torch`模块,然后创建了一个二维Tensor。`mean()`函数默认对所有元素求平均,而`dim`参数允许你选择沿着哪个轴(dimension)计算平均值。
阅读全文
相关推荐
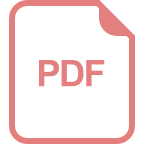
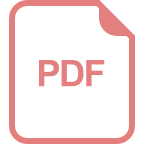
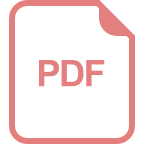













