用springboot框架写一个案例
时间: 2023-06-30 18:16:22 浏览: 98
好的,我可以给你一个简单的例子。这个例子是一个简单的RESTful API,可以用来管理学生信息。
首先,你需要在你的开发环境中安装Spring Boot框架。然后创建一个新的Spring Boot项目,添加必要的依赖项(例如Spring Web、Spring Data JPA以及MySQL数据库驱动程序等),并创建一个简单的数据模型类,例如:
```java
@Entity
@Table(name = "students")
public class Student {
@Id
@GeneratedValue(strategy = GenerationType.IDENTITY)
private Long id;
private String name;
private Integer age;
private String gender;
// getters and setters
}
```
接下来,你需要创建一个控制器类来处理HTTP请求,并将它们转换为对模型类的操作。例如:
```java
@RestController
@RequestMapping("/students")
public class StudentController {
@Autowired
private StudentRepository repository;
@GetMapping("")
public List<Student> getAllStudents() {
return repository.findAll();
}
@GetMapping("/{id}")
public Student getStudentById(@PathVariable Long id) {
return repository.findById(id).orElseThrow(() -> new ResourceNotFoundException("Student not found with id " + id));
}
@PostMapping("")
public Student createStudent(@RequestBody Student student) {
return repository.save(student);
}
@PutMapping("/{id}")
public Student updateStudent(@PathVariable Long id, @RequestBody Student studentDetails) {
Student student = repository.findById(id).orElseThrow(() -> new ResourceNotFoundException("Student not found with id " + id));
student.setName(studentDetails.getName());
student.setAge(studentDetails.getAge());
student.setGender(studentDetails.getGender());
return repository.save(student);
}
@DeleteMapping("/{id}")
public ResponseEntity<?> deleteStudent(@PathVariable Long id) {
Student student = repository.findById(id).orElseThrow(() -> new ResourceNotFoundException("Student not found with id " + id));
repository.delete(student);
return ResponseEntity.ok().build();
}
}
```
最后,你需要创建一个Spring Boot应用程序类,并在其中添加必要的配置,例如:
```java
@SpringBootApplication
public class StudentManagementApplication {
public static void main(String[] args) {
SpringApplication.run(StudentManagementApplication.class, args);
}
@Bean
public CommandLineRunner init(StudentRepository repository) {
return args -> {
repository.save(new Student(null, "Alice", 20, "Female"));
repository.save(new Student(null, "Bob", 21, "Male"));
repository.save(new Student(null, "Charlie", 22, "Male"));
};
}
@Bean
public WebMvcConfigurer corsConfigurer() {
return new WebMvcConfigurer() {
@Override
public void addCorsMappings(CorsRegistry registry) {
registry.addMapping("/**").allowedOrigins("*").allowedMethods("*").allowedHeaders("*");
}
};
}
}
```
这些配置将创建一个初始化数据的方法,并配置跨域访问。
这就是一个简单的Spring Boot案例,它可以用来管理学生信息。你可以使用Postman或其他工具来测试它的功能。
阅读全文
相关推荐
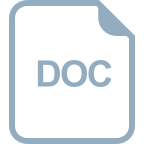
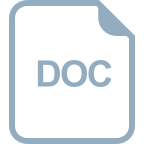
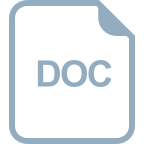
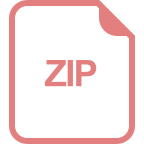
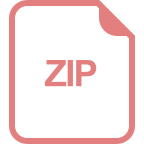
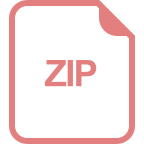
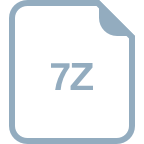
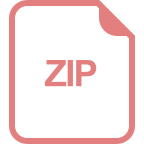
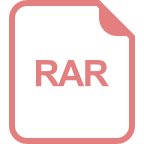
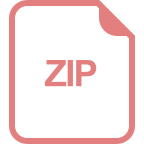
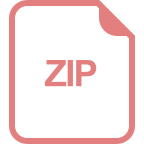
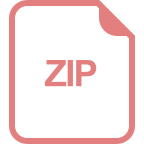
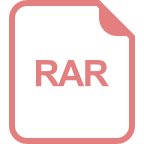
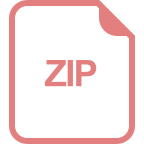
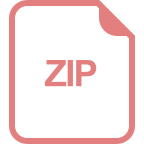
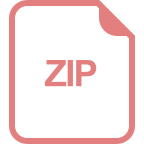
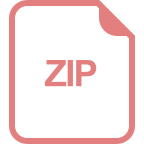
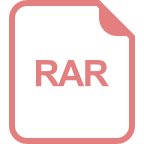