換另一種方式寫汉诺塔的非递归实现代码
时间: 2023-12-27 16:03:53 浏览: 84
以下是使用栈实现汉诺塔的非递归代码:
```python
class Stack:
def __init__(self):
self.items = []
def push(self, item):
self.items.append(item)
def pop(self):
return self.items.pop()
def is_empty(self):
return len(self.items) == 0
def move_disk(fp, tp):
print("移动盘子从", fp, "到", tp)
def hanoi_iterative(num_disks, from_peg, to_peg, aux_peg):
stack = Stack()
stack.push((num_disks, from_peg, to_peg, aux_peg))
while not stack.is_empty():
num_disks, from_peg, to_peg, aux_peg = stack.pop()
if num_disks == 1:
move_disk(from_peg, to_peg)
else:
stack.push((num_disks - 1, from_peg, aux_peg, to_peg))
stack.push((1, from_peg, to_peg, aux_peg))
stack.push((num_disks - 1, aux_peg, to_peg, from_peg))
hanoi_iterative(3, "A", "C", "B")
```
此代码中,我们使用一个栈来模拟递归调用的过程。当我们需要移动单个盘子时,我们直接调用 `move_disk` 函数。当我们需要移动多个盘子时,我们将三个操作分解为以下步骤:
1. 将前 n-1 个盘子从 from_peg 移动到 aux_peg
2. 将第 n 个盘子从 from_peg 移动到 to_peg
3. 将前 n-1 个盘子从 aux_peg 移动到 to_peg
我们将这三个步骤压入栈中,并在下一次迭代中执行它们。最后,当栈为空时,我们完成了所有的移动操作。
阅读全文
相关推荐





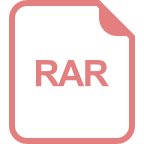



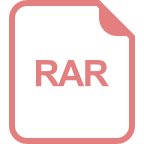

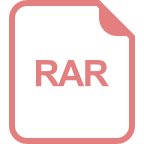


