图书管理系统中可实现书籍库存量管理的基于xml开发的代码
时间: 2024-02-22 08:59:41 浏览: 104
好的,下面是一个基于XML开发的示例代码,用来实现图书库存管理:
1. 在书籍信息XML文件中添加库存字段
在书籍信息XML文件中添加一个名为`stock`的字段,用来存放每本书的库存数量。以下是一个简单的示例:
```xml
<books>
<book>
<id>1</id>
<name>Java Programming</name>
<author>John Smith</author>
<price>29.99</price>
<stock>10</stock>
</book>
<book>
<id>2</id>
<name>Python Programming</name>
<author>Jane Doe</author>
<price>19.99</price>
<stock>5</stock>
</book>
<!-- 其他书籍信息 -->
</books>
```
2. 在借阅和归还功能中更新库存数量
在借阅和归还功能中,根据书籍ID查询对应的书籍信息,并更新库存数量。以下是一个简单的示例:
```java
// 借阅书籍
public void borrowBook(String bookId) throws Exception {
// 加载书籍信息XML文件
Document doc = XmlUtil.loadXml("books.xml");
// 根据书籍ID查询书籍信息
Node bookNode = XmlUtil.selectSingleNode(doc, "/books/book[id='" + bookId + "']");
if (bookNode == null) {
System.out.println("该书籍不存在!");
return;
}
// 获取库存数量
int stock = Integer.parseInt(XmlUtil.selectSingleNodeText(bookNode, "stock"));
if (stock <= 0) {
System.out.println("该书籍库存不足!");
return;
}
// 更新库存数量
XmlUtil.updateSingleNodeText(bookNode, "stock", String.valueOf(stock - 1));
// 保存书籍信息XML文件
XmlUtil.saveXml(doc, "books.xml");
}
// 归还书籍
public void returnBook(String bookId, int count) throws Exception {
// 加载书籍信息XML文件
Document doc = XmlUtil.loadXml("books.xml");
// 根据书籍ID查询书籍信息
Node bookNode = XmlUtil.selectSingleNode(doc, "/books/book[id='" + bookId + "']");
if (bookNode == null) {
System.out.println("该书籍不存在!");
return;
}
// 获取库存数量
int stock = Integer.parseInt(XmlUtil.selectSingleNodeText(bookNode, "stock"));
// 更新库存数量
XmlUtil.updateSingleNodeText(bookNode, "stock", String.valueOf(stock + count));
// 保存书籍信息XML文件
XmlUtil.saveXml(doc, "books.xml");
}
```
以上示例代码中,我们使用了一个名为`XmlUtil`的工具类来操作XML文件。在借阅和归还功能中,首先加载书籍信息XML文件,然后根据书籍ID查询对应的书籍信息,并获取库存数量。在借阅时,如果库存数量不足,则不能借阅;在归还时,需要将归还的数量加上原有的库存数量,然后更新库存数量并保存到书籍信息XML文件中。
阅读全文
相关推荐

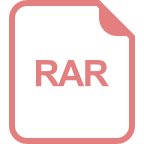



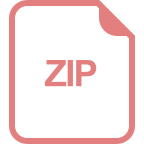




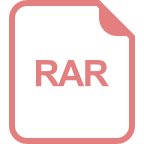





