opencv 进行对point2f 线条进行 与 或 运算
时间: 2023-12-10 14:37:54 浏览: 23
在OpenCV中,Point2f是一个表示二维平面上点的数据类型,而线条可以用LineIterator类表示。但是,Point2f和LineIterator之间是没有逻辑运算的。
如果您想对二维平面上的点进行逻辑运算,可以使用Mat类和相应的位运算函数,例如bitwise_or()和bitwise_and()函数。具体地说,您可以将每个点表示为二维坐标系中的像素,将它们存储在一个Mat对象中,并使用bitwise_or()或bitwise_and()函数对它们进行逻辑运算。例如:
```
Mat mask1 = Mat::zeros(height, width, CV_8UC1);
Mat mask2 = Mat::zeros(height, width, CV_8UC1);
// 在mask1和mask2中分别设置一些点
// ...
Mat result;
bitwise_or(mask1, mask2, result); // 对mask1和mask2进行逻辑或运算
```
如果您想对线条进行逻辑运算,则需要首先将线条表示为二进制图像,然后使用上述方法进行逻辑运算。
相关问题
opencv 怎么进行point2f 数据 平移
要对 Point2f 进行平移,可以使用 OpenCV 中的函数 `cv::Point2f::operator+=()` 和 `cv::Point2f::operator-=()`。
举个例子,如果你想将一个 Point2f 对象 `p` 沿着 x 轴平移 `dx`,沿着 y 轴平移 `dy`,可以这样做:
```
cv::Point2f p(1.0, 2.0);
float dx = 0.5;
float dy = -0.5;
p += cv::Point2f(dx, dy);
```
这里,我们创建了一个 Point2f 对象 `p`,其初始坐标为 (1.0, 2.0)。然后,我们定义了两个浮点数 `dx` 和 `dy`,分别表示 x 和 y 方向上的平移量。最后,我们使用 `+=` 运算符将 `p` 沿着指定方向平移。
如果要将 `p` 往相反的方向平移,可以使用 `-=` 运算符:
```
p -= cv::Point2f(dx, dy);
```
这样,`p` 的坐标就会回到初始状态了。
OpenCvSharp中Point2f转换成InputArray
在OpenCvSharp中,Point2f可以直接转换为InputArray类型的变量,而不需要进行额外的转换。InputArray是OpenCV中的一个通用类型,用于表示输入数据,并可以接受多种类型的输入,如Mat、std::vector等。
以下是一个示例代码,演示如何将Point2f转换为InputArray:
```csharp
using OpenCvSharp;
// 输入参数:点坐标
public void ProcessPoint(Point2f point)
{
// 将Point2f转换为InputArray类型的变量
InputArray input = InputArray.Create(new Point2f[] { point });
// 在输入数据上执行某些操作...
// ...
}
```
在上面的代码中,我们把Point2f数组包装成一个InputArray对象,然后可以将其作为输入数据传递给需要的函数,例如在图像中绘制点的函数。
需要注意的是,InputArray是一个只读类型,这意味着它不能被修改。如果需要修改输入数据,请使用Mat或其他可写类型的变量。
相关推荐
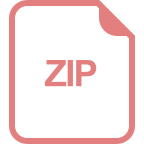












