请帮用flask和mysql生成一套学生管理系统的代码,实现系统登录,学生信息的增删改查功能。请生成python代码和html代码哦
时间: 2024-05-02 11:17:29 浏览: 12
好的,以下是使用 Flask 和 MySQL 实现学生管理系统的 Python 代码和 HTML 代码。
Python 代码:
```python
from flask import Flask, render_template, request, redirect, url_for
import pymysql
app = Flask(__name__)
app.config['SECRET_KEY'] = 'secret_key'
# MySQL连接配置
conn = pymysql.connect(host='localhost', port=3306, user='root', passwd='password', db='db_name', charset='utf8')
# 登录页面
@app.route('/', methods=['GET', 'POST'])
def login():
if request.method == 'POST':
username = request.form['username']
password = request.form['password']
cursor = conn.cursor()
cursor.execute("SELECT * FROM users WHERE username=%s AND password=%s", (username, password))
user = cursor.fetchone()
if user:
return redirect(url_for('students'))
else:
return render_template('login.html', error='用户名或密码错误')
else:
return render_template('login.html')
# 学生列表页面
@app.route('/students')
def students():
cursor = conn.cursor()
cursor.execute("SELECT * FROM students")
students = cursor.fetchall()
return render_template('students.html', students=students)
# 添加学生页面
@app.route('/add_student', methods=['GET', 'POST'])
def add_student():
if request.method == 'POST':
name = request.form['name']
age = request.form['age']
sex = request.form['sex']
department = request.form['department']
cursor = conn.cursor()
cursor.execute("INSERT INTO students(name, age, sex, department) VALUES(%s, %s, %s, %s)", (name, age, sex, department))
conn.commit()
return redirect(url_for('students'))
else:
return render_template('add_student.html')
# 修改学生页面
@app.route('/edit_student/<int:id>', methods=['GET', 'POST'])
def edit_student(id):
if request.method == 'POST':
name = request.form['name']
age = request.form['age']
sex = request.form['sex']
department = request.form['department']
cursor = conn.cursor()
cursor.execute("UPDATE students SET name=%s, age=%s, sex=%s, department=%s WHERE id=%s", (name, age, sex, department, id))
conn.commit()
return redirect(url_for('students'))
else:
cursor = conn.cursor()
cursor.execute("SELECT * FROM students WHERE id=%s", id)
student = cursor.fetchone()
return render_template('edit_student.html', student=student)
# 删除学生
@app.route('/delete_student/<int:id>')
def delete_student(id):
cursor = conn.cursor()
cursor.execute("DELETE FROM students WHERE id=%s", id)
conn.commit()
return redirect(url_for('students'))
if __name__ == '__main__':
app.run()
```
HTML 代码:
`login.html`
```html
<!DOCTYPE html>
<html>
<head>
<meta charset="UTF-8">
<title>学生管理系统 - 登录</title>
</head>
<body>
<h1>学生管理系统 - 登录</h1>
{% if error %}
<p style="color: red;">{{ error }}</p>
{% endif %}
<form method="post">
<label>用户名:</label>
<input type="text" name="username" required><br><br>
<label>密码:</label>
<input type="password" name="password" required><br><br>
<input type="submit" value="登录">
</form>
</body>
</html>
```
`students.html`
```html
<!DOCTYPE html>
<html>
<head>
<meta charset="UTF-8">
<title>学生管理系统 - 学生列表</title>
</head>
<body>
<h1>学生管理系统 - 学生列表</h1>
<a href="/add_student">添加学生</a><br><br>
<table border="1">
<tr>
<th>ID</th>
<th>姓名</th>
<th>年龄</th>
<th>性别</th>
<th>系别</th>
<th>操作</th>
</tr>
{% for student in students %}
<tr>
<td>{{ student[0] }}</td>
<td>{{ student[1] }}</td>
<td>{{ student[2] }}</td>
<td>{{ student[3] }}</td>
<td>{{ student[4] }}</td>
<td>
<a href="/edit_student/{{ student[0] }}">修改</a>
<a href="/delete_student/{{ student[0] }}" onclick="return confirm('确定要删除吗?')">删除</a>
</td>
</tr>
{% endfor %}
</table>
</body>
</html>
```
`add_student.html`
```html
<!DOCTYPE html>
<html>
<head>
<meta charset="UTF-8">
<title>学生管理系统 - 添加学生</title>
</head>
<body>
<h1>学生管理系统 - 添加学生</h1>
<form method="post">
<label>姓名:</label>
<input type="text" name="name" required><br><br>
<label>年龄:</label>
<input type="number" name="age" required><br><br>
<label>性别:</label>
<input type="radio" name="sex" value="男" checked>男
<input type="radio" name="sex" value="女">女<br><br>
<label>系别:</label>
<input type="text" name="department" required><br><br>
<input type="submit" value="添加">
</form>
<br>
<a href="/students">返回学生列表</a>
</body>
</html>
```
`edit_student.html`
```html
<!DOCTYPE html>
<html>
<head>
<meta charset="UTF-8">
<title>学生管理系统 - 修改学生</title>
</head>
<body>
<h1>学生管理系统 - 修改学生</h1>
<form method="post">
<label>姓名:</label>
<input type="text" name="name" value="{{ student[1] }}" required><br><br>
<label>年龄:</label>
<input type="number" name="age" value="{{ student[2] }}" required><br><br>
<label>性别:</label>
<input type="radio" name="sex" value="男" {% if student[3] == '男' %}checked{% endif %}>男
<input type="radio" name="sex" value="女" {% if student[3] == '女' %}checked{% endif %}>女<br><br>
<label>系别:</label>
<input type="text" name="department" value="{{ student[4] }}" required><br><br>
<input type="submit" value="修改">
</form>
<br>
<a href="/students">返回学生列表</a>
</body>
</html>
```
注意:需要根据实际情况修改 MySQL 连接配置和数据库表结构。
相关推荐
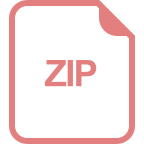
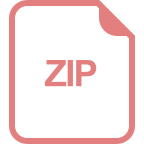














