python datetime 如何得到年月日十分秒和毫秒
时间: 2023-08-31 22:21:35 浏览: 192
### 回答1:
你可以使用 `datetime` 模块中的 `datetime` 类的以下属性来获取年月日时分秒和毫秒:
- year: 年份
- month: 月份
- day: 日期
- hour: 小时
- minute: 分钟
- second: 秒
- microsecond: 微秒
例如:
```python
import datetime
now = datetime.datetime.now()
print(now.year)
print(now.month)
print(now.day)
print(now.hour)
print(now.minute)
print(now.second)
print(now.microsecond)
```
运行上面的代码将会输出当前时间的年月日时分秒和毫秒。
注意: 如果你希望得到格式化的日期字符串, 你可以使用 `strftime` 方法。例如:
```python
formatted_date = now.strftime('%Y-%m-%d %H:%M:%S')
print(formatted_date)
```
这将会输出类似于 "2022-01-07 15:30:00" 的字符串。
### 回答2:
在使用Python的datetime模块中,要获取年、月、日、小时、分钟、秒以及毫秒,可以使用以下方法:
1. 获取年、月、日:
```
import datetime
now = datetime.datetime.now()
year = now.year
month = now.month
day = now.day
print(year, month, day)
```
这将打印出当前时间的年、月、日。
2. 获取小时、分钟、秒:
```
import datetime
now = datetime.datetime.now()
hour = now.hour
minute = now.minute
second = now.second
print(hour, minute, second)
```
这将打印出当前时间的小时、分钟、秒。
3. 获取毫秒:
```
import datetime
now = datetime.datetime.now()
millisecond = now.microsecond // 1000
print(millisecond)
```
这将打印出当前时间的毫秒(注意:datetime模块的microsecond属性表示微秒,通过除以1000得到毫秒)。
综上所述,以上代码演示了如何使用datetime模块获取年、月、日、小时、分钟、秒和毫秒的方法。
### 回答3:
在Python中,可以使用datetime模块来获取年、月、日、小时、分钟、秒和毫秒。首先,需要导入datetime模块。
```python
import datetime
```
然后,可以使用datetime模块的now()函数来获取当前日期和时间的datetime对象。
```python
now = datetime.datetime.now()
```
接下来,可以使用datetime对象的属性来获取具体的年、月、日、小时、分钟、秒和毫秒。
```python
year = now.year # 年
month = now.month # 月
day = now.day # 日
hour = now.hour # 小时
minute = now.minute # 分钟
second = now.second # 秒
microsecond = now.microsecond # 毫秒
```
要注意的是,毫秒的值是一个整数,表示当前时间的微秒数。如果想要获取到毫秒的数值,可以通过除以1000来得到。
```python
millisecond = now.microsecond // 1000 # 毫秒
```
最后,可以根据需要打印出获取到的年、月、日、小时、分钟、秒和毫秒的数值。
```python
print("年:", year)
print("月:", month)
print("日:", day)
print("小时:", hour)
print("分钟:", minute)
print("秒:", second)
print("毫秒:", millisecond)
```
这样就能够得到Python中datetime对象的年、月、日、小时、分钟、秒和毫秒的数值了。
阅读全文
相关推荐
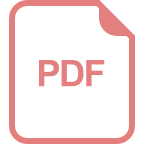
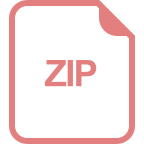


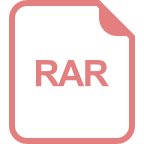











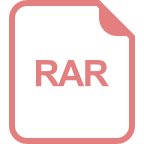
