在 Microsoft Visual Studio 的 win32控制台程序中使用C语言写一个定时器,设置为1500ms溢出,并封装成函数。
时间: 2024-10-13 22:06:17 浏览: 29
在Microsoft Visual Studio的Win32控制台程序中使用C语言创建一个定时器,通常我们会利用Windows API中的`SetTimer`函数配合`CreateThread`来实现实时任务调度。然而,Windows API并不直接提供1500ms的定时器,所以我们需要通过某种间接的方式来实现这个需求。
首先,你需要包含必要的头文件,如`windows.h`:
```c
#include <windows.h>
```
然后,我们可以定义一个函数,例如`start_timer`,它接受一个回调函数作为参数,该回调函数将在定时器溢出时被调用:
```c
typedef void (WINAPI *TIMER_CALLBACK)(unsigned long id, unsigned long elapsed);
BOOL start_timer(unsigned long interval_ms, TIMER_CALLBACK callback)
{
static const DWORD CALLBACK timer_proc(LPVOID lpParam)
{
if (!lpParam) return TRUE; // 假设callback未传递时结束
unsigned long elapsed = GetTickCount();
callback((unsigned long)-1, elapsed); // 函数参数可能需调整
return SetTimer(NULL, (TIMER_ID)lpParam, interval_ms, timer_proc);
}
HANDLE hTimer = CreateThread(NULL, 0, timer_proc, (LPVOID)&interval_ms, 0, NULL);
if (hTimer == INVALID_HANDLE_VALUE)
{
// 错误处理,如添加日志
return FALSE;
}
else
{
return TRUE;
}
}
```
在这个例子中,我们创建了一个新的线程来持续执行定时任务,`timer_proc`是一个回调函数,每间隔`interval_ms`毫秒就会被调用一次。当`GetTickCount()`返回值超过上次的值时,表示时间已过期。
为了停止定时器,你可以传递一个特定的标识符给`StopTimer`或在`timer_proc`中添加适当的条件检查来关闭定时器:
```c
void stop_timer(unsigned long timer_id)
{
KillTimer(NULL, timer_id);
}
```
阅读全文
相关推荐
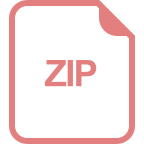
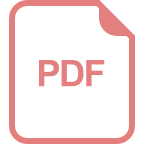
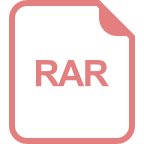



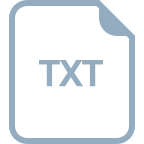
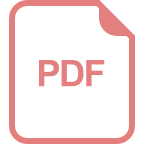
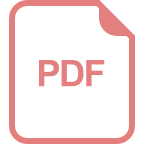
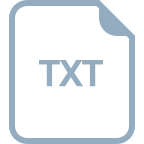
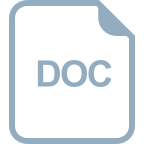
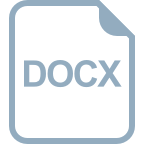
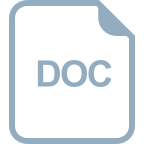
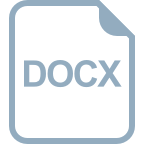
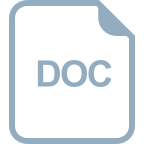
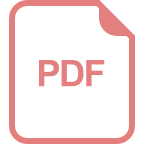