Java实现短作业优先算法,PCB里需要有进程名,进程状态,运行时间和到达时间以及链表
时间: 2024-03-17 10:42:55 浏览: 25
好的,以下是一个简单的Java实现短作业优先算法的例子,其中PCB类包含了进程名、进程状态、运行时间和到达时间等属性,以及一个链表用来存储下一个进程:
```java
import java.util.*;
class PCB {
String name;
String status;
int runTime;
int arriveTime;
PCB next;
public PCB(String name, int runTime, int arriveTime) {
this.name = name;
this.runTime = runTime;
this.arriveTime = arriveTime;
this.status = "ready";
this.next = null;
}
public String toString() {
return name + " " + status + " " + runTime + " " + arriveTime;
}
}
public class SJF {
public static void main(String[] args) {
PCB head = new PCB("P1", 3, 0);
head.next = new PCB("P2", 2, 1);
head.next.next = new PCB("P3", 1, 2);
head.next.next.next = new PCB("P4", 4, 3);
int currentTime = 0;
while (head != null) {
// 选择当前可运行的进程中预计运行时间最短的进程
PCB shortestJob = head;
PCB prev = null;
PCB current = head;
while (current != null) {
if (current.status.equals("ready") && current.arriveTime <= currentTime && current.runTime < shortestJob.runTime) {
shortestJob = current;
prev = prev;
}
prev = current;
current = current.next;
}
if (shortestJob != null) {
shortestJob.status = "running";
currentTime += shortestJob.runTime;
shortestJob.runTime = 0;
shortestJob.status = "finished";
if (prev == null) {
head = shortestJob.next;
} else {
prev.next = shortestJob.next;
}
} else {
currentTime++;
}
}
System.out.println("All processes finished!");
}
}
```
在该例子中,我们创建了一个PCB类来存储进程信息,其中包括进程名、进程状态、运行时间和到达时间等属性,以及一个链表用来存储下一个进程。在主函数中,我们创建了一个PCB链表,并使用一个while循环来模拟整个进程调度过程,每次选择当前可运行的进程中预计运行时间最短的进程进行调度。在每次调度完成后,我们更新当前时间、更新进程状态,并在链表中删除已完成的进程。最终,当链表为空时,我们输出所有进程已完成的信息。
相关推荐
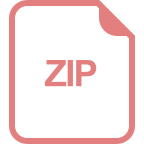
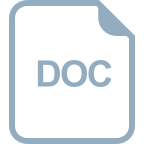
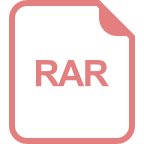














