c语言一个排序好的数组,要求输入一个数按照原数组的排序方式进行排序
时间: 2023-06-12 21:04:23 浏览: 46
可以使用插入排序的思想,从原数组的第一个元素开始,逐个比较输入的数与原数组中的数的大小关系,找到要插入的位置,然后将原数组中的元素后移一位,最后将输入的数插入到该位置即可。
以下是示例代码:
```c
#include <stdio.h>
#define MAX_SIZE 100
void insert(int arr[], int n, int x);
int main() {
int arr[MAX_SIZE], n, x, i;
// 读入原数组
printf("Enter the size of the array: ");
scanf("%d", &n);
printf("Enter the elements of the array in ascending order:\n");
for (i = 0; i < n; i++) {
scanf("%d", &arr[i]);
}
// 读入要插入的数
printf("Enter the number to be inserted: ");
scanf("%d", &x);
// 插入数并输出结果
insert(arr, n, x);
printf("The array after inserting %d:\n", x);
for (i = 0; i <= n; i++) {
printf("%d ", arr[i]);
}
return 0;
}
void insert(int arr[], int n, int x) {
int i, j;
// 找到要插入的位置
for (i = 0; i < n; i++) {
if (x < arr[i]) {
break;
}
}
// 将原数组中的元素后移一位
for (j = n; j > i; j--) {
arr[j] = arr[j-1];
}
// 插入数
arr[i] = x;
}
```
注意,上述代码没有考虑输入的数比原数组中所有数都大的情况,需要根据具体情况进行修改。
相关推荐
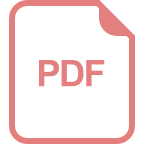
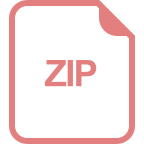
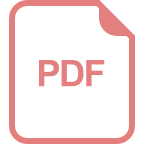














