python如何将记事本中的多行文件读取出来
时间: 2024-02-18 20:00:10 浏览: 131
Python 中可以使用 `readlines()` 方法将一个文本文件中的多行文本读取为一个列表。具体步骤如下:
1. 打开一个文本文件,使用 `readlines()` 方法读取多行文本。
2. 对于每一行的文本,可以使用字符串方法(如 `strip()`)去除行末的空白字符。
3. 关闭文件。
以下是示例代码:
```python
with open('test.txt', 'r') as file:
lines = file.readlines() # 读取多行文本
# 对于每一行的文本,使用 strip() 方法去除行末的空白字符
lines = [line.strip() for line in lines]
print(lines) # 打印读取出的多行文本
# 关闭文件
file.close()
```
在上面的代码中,我们使用 `with` 语句打开文件,这样可以自动关闭文件,不需要显式地调用 `close()` 方法。如果你需要在文件操作结束后手动关闭文件,可以使用 `file.close()` 方法。
相关问题
python中如何读取多行excel数据
可以使用 pandas 库来读取多行 Excel 数据。具体代码如下:
```
import pandas as pd
df = pd.read_excel("文件名.xlsx", sheet_name="工作表名"
header=0, nrows=10, usecols=[0, 3, 5])
```
其中,`"文件名.xlsx"` 为要读取的 Excel 文件名,`"工作表名"` 为要读取的工作表名。`header=0` 表示指定使用第一行作为表头,`nrows=10` 表示只读取前十行,`usecols=[0, 3, 5]` 表示只读取第一、四、六列的数据。最后读取到的数据保存在 df 变量中。
python读取csv文件多行代码示例
Here is an example of reading multiple lines from a CSV file in Python:
```
import csv
with open('data.csv', 'r') as file:
reader = csv.reader(file)
for row in reader:
print(row)
```
This code will read the `data.csv` file and print each row as a list of values. The `csv.reader` function is used to create a reader object that can be used to iterate over the rows in the file. The `with` statement is used to ensure that the file is properly closed after it has been read.
阅读全文
相关推荐
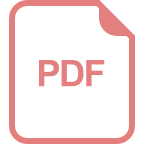
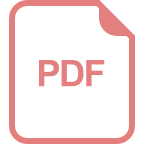
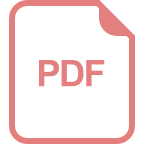













