Qt编写简单Object-C代码
时间: 2024-01-15 19:02:45 浏览: 64
下面是一个简单的Objective-C++代码示例,可以在Qt中使用:
```objective-c
#include <QCoreApplication>
#include <QProcess>
int main(int argc, char *argv[])
{
QCoreApplication a(argc, argv);
QProcess process;
process.start("osascript", QStringList() << "-e" << "display dialog \"Hello from Objective-C!\"");
process.waitForFinished(-1);
return a.exec();
}
```
这个代码使用了Qt的QProcess类来执行Objective-C++代码。它调用了osascript命令,并且传递了一个参数来显示一个对话框。当osascript命令执行完成后,程序将会退出。
相关问题
使用Qt编写的代码,可以显示电脑本机安装的全部软件的图标和名称
可以使用Qt的QProcess类来执行系统命令,然后解析命令输出的结果来获取安装的软件信息。
以下是一个简单的示例程序,它使用QProcess类来执行Windows系统命令,获取安装的软件信息,并将软件图标和名称显示在Qt的ListView控件中:
```cpp
#include <QApplication>
#include <QListView>
#include <QStandardItemModel>
#include <QProcess>
#include <QFileIconProvider>
int main(int argc, char *argv[])
{
QApplication app(argc, argv);
// 创建ListView控件和数据模型
QListView listView;
QStandardItemModel model;
listView.setModel(&model);
listView.setIconSize(QSize(32, 32));
// 创建文件图标提供者
QFileIconProvider iconProvider;
// 执行系统命令获取安装的软件信息
QProcess process;
process.start("powershell Get-WmiObject Win32_Product | Select-Object Name, InstallLocation");
process.waitForFinished();
QString output = process.readAllStandardOutput();
// 解析命令输出的结果,并将软件图标和名称添加到数据模型中
QStringList lines = output.split('\n');
for (QString line : lines) {
line = line.trimmed();
if (line.isEmpty()) {
continue;
}
QStringList fields = line.split(" ", QString::SkipEmptyParts);
if (fields.size() != 2) {
continue;
}
QString name = fields[0];
QString path = fields[1];
QString iconPath = iconProvider.icon(QFileInfo(path)).pixmap(32, 32).toImage().save(name + ".png") ? name + ".png" : "";
QStandardItem *item = new QStandardItem(iconProvider.icon(QFileInfo(iconPath)), name);
model.appendRow(item);
}
// 显示ListView控件
listView.show();
return app.exec();
}
```
这个示例程序使用了Windows系统自带的PowerShell命令行工具来获取安装的软件信息,然后使用Qt的QFileIconProvider类来获取每个软件的图标,最后将软件图标和名称添加到Qt的数据模型中,并显示在ListView控件中。
需要注意的是,由于这个程序使用了系统命令行工具来获取安装的软件信息,所以它只适用于Windows系统。如果需要在其他操作系统上运行,需要使用相应的命令行工具来获取软件信息。
python qt 将QGraphicsView对象传入qt编写的dll中
将QGraphicsView对象传入Qt编写的DLL中需要以下步骤:
1. 在Qt编写的DLL中定义一个函数,该函数的参数包括QGraphicsView对象和其他需要的参数。
2. 在Python中通过ctypes库加载Qt编写的DLL,获取函数并调用。
3. 在Python中创建QGraphicsView对象,将其作为参数传递给Qt编写的DLL中的函数。
下面是一个简单的示例代码:
Qt编写的DLL中的函数:
```cpp
#include <QGraphicsView>
#ifdef Q_OS_WIN
#define EXPORT __declspec(dllexport)
#else
#define EXPORT
#endif
extern "C"
{
EXPORT void process(QGraphicsView* view, int param1, float param2)
{
// do something with the view and other parameters
}
}
```
Python中的代码:
```python
import ctypes
from PyQt5.QtWidgets import QGraphicsView
# load the DLL
mydll = ctypes.cdll.LoadLibrary("mydll.dll")
# get the function
process = mydll.process
process.argtypes = [ctypes.POINTER(QGraphicsView), ctypes.c_int, ctypes.c_float]
# create a QGraphicsView object
view = QGraphicsView()
# call the function with the view object and other parameters
process(ctypes.pointer(view), 42, 3.14)
```
注意事项:
1. 在Qt编写的DLL中,需要使用extern "C"将函数声明为C风格的函数,以便Python能够正确地调用它。
2. 在Python中,需要使用ctypes.POINTER将QGraphicsView对象转换为指针类型,并将其作为参数传递给函数。
阅读全文
相关推荐
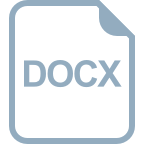
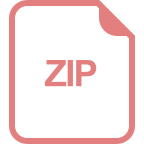
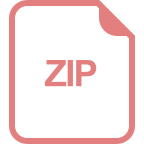
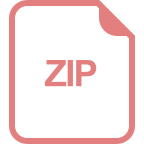
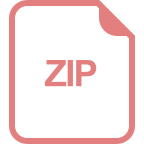
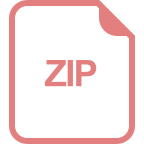
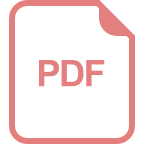
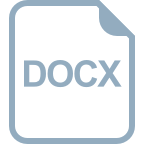


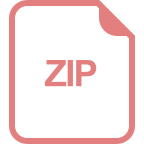

