#include <iostream> using namespace std; class Date { public: Date(int y, int m, int d) { year = y; months = m; date = d; } ~Date() { } protected: int year; int months; int date; }; class Time :public Date { public: Time(int y, int m, int d, int h, int mi, int s) :Date(y, m, d) { hour = h; min = mi; sec = s; } void display() { int a = sec + 1; cout << "年:" << year << endl; cout << "月:" << months << endl; cout << "日:" << date << endl; cout << "时:" << hour << endl; cout << "分:" << min << endl; cout << "秒:" << a << endl; } ~Time(){ } private: int hour; int min; int sec; }; int main() { Time time1(2003, 8, 12, 7, 34, 23); time1.display(); return 0;优化这段代码
时间: 2024-01-27 08:03:43 浏览: 107
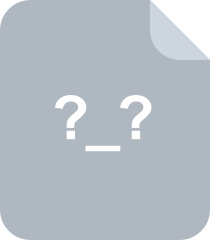
#include <iostream>
这段代码已经相对简洁明了了,但还是可以进行一些优化。以下是我提出的优化建议:
1. 使用初始化列表来初始化成员变量,而不是在构造函数中进行赋值操作。这样可以提高代码的执行效率。
2. 将析构函数定义为虚函数,以便于继承。
3. 将类的成员变量定义为私有的,通过公共的接口函数来访问和修改成员变量的值,这样可以提高代码的安全性。
4. 在输出时,可以使用"\n"代替endl,以提高代码的执行效率。
下面是优化后的代码:
```
#include <iostream>
using namespace std;
class Date {
public:
Date(int y, int m, int d) : year(y), months(m), date(d) {}
virtual ~Date() {}
int getYear() const { return year; }
int getMonth() const { return months; }
int getDate() const { return date; }
private:
int year;
int months;
int date;
};
class Time : public Date {
public:
Time(int y, int m, int d, int h, int mi, int s) : Date(y, m, d), hour(h), min(mi), sec(s) {}
virtual ~Time(){}
int getHour() const { return hour; }
int getMin() const { return min; }
int getSec() const { return sec; }
void display() const {
int a = sec + 1;
cout << "年:" << getYear() << "\n";
cout << "月:" << getMonth() << "\n";
cout << "日:" << getDate() << "\n";
cout << "时:" << hour << "\n";
cout << "分:" << min << "\n";
cout << "秒:" << a << "\n";
}
private:
int hour;
int min;
int sec;
};
int main() {
Time time1(2003, 8, 12, 7, 34, 23);
time1.display();
return 0;
}
```
阅读全文
相关推荐
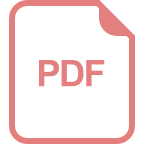
















